return F.conv3d( RuntimeError: expected scalar type Byte but found Float
时间: 2023-10-29 07:55:51 浏览: 258
在你的问题中,你提到了一个错误信息:RuntimeError: expected scalar type Byte but found Float。这个错误通常是由于输入的张量类型与期望的类型不匹配导致的。根据你提供的引用,可能的解决方法是将输入的张量类型转换为期望的类型。你可以尝试使用`.to()`方法将张量转换为Byte类型,如下所示:
```python
input_tensor = input_tensor.to(torch.ByteTensor)
```
这将把`input_tensor`转换为Byte类型的张量,以匹配期待的类型。然后,你可以再次运行`return F.conv3d()`函数,看看是否解决了错误。
相关问题
return F.conv3d( RuntimeError: expected scalar type Double but found Float
This error message indicates that there is a mismatch between the data type of the input tensor and the expected data type by the convolutional layer. The convolutional layer expects the input tensor to be of data type double, but it is finding data type float instead.
To resolve this error, you can convert the input tensor to double data type before passing it to the convolutional layer. You can use the `.double()` function to convert the tensor to double data type. For example:
```python
input_tensor = input_tensor.double()
output_tensor = F.conv3d(input_tensor, weight_tensor, ...)
```
Alternatively, you can modify the convolutional layer to accept float data type as input by specifying the `dtype` argument. For example:
```python
output_tensor = F.conv3d(input_tensor, weight_tensor, ..., dtype=torch.float32)
```
Note that you need to modify the `dtype` argument to match the data type of your input tensor.
RuntimeError: expected scalar type Double but found Float
这个错误通常发生在使用 PyTorch 时,因为 PyTorch 中默认的数据类型是 FloatTensor,而有些模型需要的是 DoubleTensor 类型的数据。解决这个问题的方法是将数据类型转换为 DoubleTensor,可以使用以下代码:
```python
x = x.double() # 将 x 转换为 DoubleTensor 类型
```
另外,也可以在模型定义时明确指定数据类型为 DoubleTensor,例如:
```python
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3)
self.fc1 = nn.Linear(64*30*30, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = self.conv1(x)
x = F.relu(x)
x = x.view(-1, 64*30*30)
x = self.fc1(x)
x = F.relu(x)
x = self.fc2(x)
return x.double() # 将输出结果转换为 DoubleTensor 类型
```
阅读全文
相关推荐











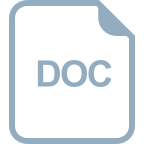

