mybatisplus购物车编写代码
时间: 2023-08-27 19:22:50 浏览: 55
当使用MyBatis Plus编写购物车功能时,您可以按照以下步骤进行操作:
1. 创建购物车表
首先,您需要创建一个购物车表来存储购物车中的商品信息。可以使用以下SQL语句创建购物车表:
```sql
CREATE TABLE shopping_cart (
id INT PRIMARY KEY AUTO_INCREMENT,
user_id INT,
product_id INT,
quantity INT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
updated_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
FOREIGN KEY (user_id) REFERENCES users(id),
FOREIGN KEY (product_id) REFERENCES products(id)
);
```
2. 创建实体类
根据购物车表的结构,创建一个购物车实体类。可以使用类似以下代码创建购物车实体类:
```java
import com.baomidou.mybatisplus.annotation.TableName;
@TableName("shopping_cart")
public class ShoppingCart {
private Long id;
private Long userId;
private Long productId;
private Integer quantity;
// getters and setters
}
```
3. 创建Mapper接口
创建一个Mapper接口来操作购物车表。可以使用以下代码作为参考:
```java
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface ShoppingCartMapper extends BaseMapper<ShoppingCart> {
// 可以在需要的情况下自定义查询方法
}
```
4. 使用Mapper进行CRUD操作
在您的服务类或控制器中,注入`ShoppingCartMapper`对象,并使用它进行CRUD操作。可以使用以下代码示例:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class ShoppingCartService {
private final ShoppingCartMapper shoppingCartMapper;
@Autowired
public ShoppingCartService(ShoppingCartMapper shoppingCartMapper) {
this.shoppingCartMapper = shoppingCartMapper;
}
// 添加商品到购物车
public void addToCart(ShoppingCart shoppingCart) {
shoppingCartMapper.insert(shoppingCart);
}
// 更新购物车中的商品数量
public void updateQuantity(ShoppingCart shoppingCart) {
shoppingCartMapper.updateById(shoppingCart);
}
// 从购物车中删除商品
public void deleteFromCart(Long cartId) {
shoppingCartMapper.deleteById(cartId);
}
// 根据用户ID查询购物车列表
public List<ShoppingCart> getCartByUserId(Long userId) {
QueryWrapper<ShoppingCart> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("user_id", userId);
return shoppingCartMapper.selectList(queryWrapper);
}
}
```
请根据您的具体业务需求进行相应的调整和扩展。这只是一个简单的示例,您可以根据实际情况进行更多的操作和逻辑处理。
相关推荐
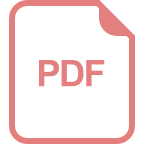














