用c语言写一篇图像旋转的代码
时间: 2024-09-10 16:25:58 浏览: 83
在C语言中编写图像旋转的代码需要对图形处理有一定的了解。图像旋转通常涉及到图像数据的读取、转换和写入。以下是一个简化的例子,展示了如何在C语言中对位图(BMP)图像进行旋转。请注意,这个例子仅用于教学目的,没有进行详细的错误检查和优化。
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265
// 用于读取和存储像素数据的结构体
typedef struct {
unsigned char* image; // 像素数据数组
int width; // 图像宽度
int height; // 图像高度
} Bitmap;
// 旋转图像函数
void rotateImage(Bitmap* image, double angle) {
// 计算图像中心
double centerX = image->width / 2;
double centerY = image->height / 2;
// 创建一个新的位图来存放旋转后的图像
Bitmap rotated;
rotated.image = malloc(image->width * image->height * sizeof(unsigned char));
rotated.width = image->width;
rotated.height = image->height;
for (int y = 0; y < image->height; y++) {
for (int x = 0; x < image->width; x++) {
// 计算旋转后的坐标
int newX = (int)(cos(angle) * (x - sin(angle) * (y - centerY) + centerX);
int newY = (int)(sin(angle) * (x - centerX) + cos(angle) * (y - centerY) + centerY);
// 确保坐标在图像范围内
if (newX >= 0 && newX < image->width && newY >= 0 && newY < image->height) {
int index = (newY * image->width + newX) * sizeof(unsigned char);
rotated.image[(y * image->width + x) * sizeof(unsigned char)] = image->image[index];
} else {
rotated.image[(y * image->width + x) * sizeof(unsigned char)] = 0; // 使用透明或其他颜色填充超出范围的部分
}
}
}
// 将旋转后的图像数据复制回原图像
free(image->image);
image->image = rotated.image;
}
int main() {
// 这里应该包含读取BMP图像文件的代码,填充image结构体
// Bitmap image = loadBMP("path_to_image.bmp");
// 假设image已经被正确加载
// Bitmap image = { ... };
// 旋转图像45度
rotateImage(&image, 45 * PI / 180);
// 这里应该包含将旋转后的图像保存到文件的代码
// saveBMP(&image, "path_to_output_image.bmp");
return 0;
}
// 这里应该定义loadBMP和saveBMP函数来处理图像的读取和保存
```
这个代码片段是一个非常基础的旋转算法,它没有考虑图像的颜色深度、调色板、压缩格式等复杂因素。在实际应用中,通常需要使用图像处理库如libpng、libjpeg或OpenCV等,这些库提供了更完善的功能来处理图像的读取、旋转以及其他操作。
阅读全文
相关推荐
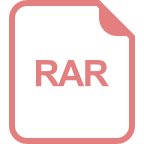
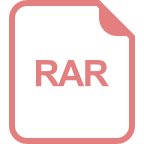
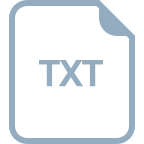
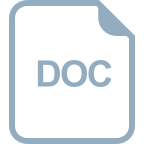
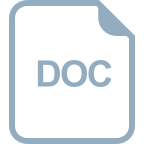
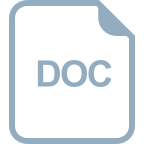

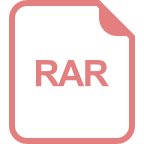
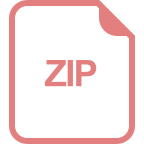
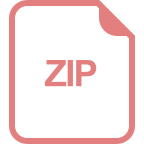
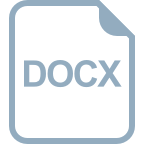
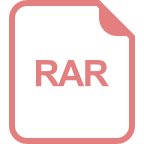
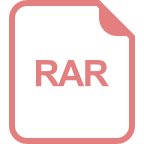
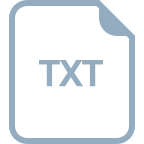
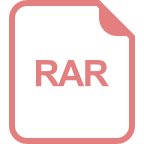
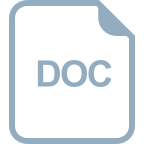
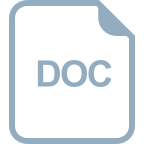
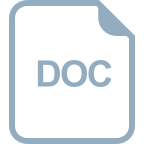
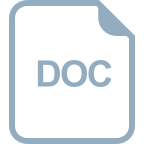