五维卡尔曼滤波C语言
时间: 2025-01-07 18:08:48 浏览: 1
### 关于五维卡尔曼滤波的C语言实现
#### 三维到多维扩展基础理论
在讨论五维卡尔曼滤波之前,先理解如何从低维度向高维度过渡。对于任何维度的状态空间模型来说,核心在于定义合适的状态转移矩阵\( \mathbf{F} \),观测矩阵 \( \mathbf{H} \),以及处理噪声的相关参数\[1\]。
#### 定义五维状态变量
假设要跟踪的对象具有五个自由度的位置和速度信息,则可以构建如下形式的状态向量:
```c
typedef struct {
double pos_x; /* Position X */
double vel_x; /* Velocity X */
double pos_y; /* Position Y */
double vel_y; /* Velocity Y */
double heading; /* Heading angle (orientation) */
} StateVector;
```
此结构体表示了一个拥有两个方向上的位置与速度分量加上航向角的状态矢量[^3]。
#### 初始化预测阶段所需的数据结构
为了完成一次完整的迭代计算,还需要初始化一些辅助性的数据结构来保存估计误差协方差P、过程噪音Q、测量噪音R等重要参数:
```c
/* Prediction stage variables initialization */
double P[5][5]; // Error covariance matrix
double Q[5][5]; // Process noise covariance matrix
double F[5][5]; // State transition model
// ... other necessary initializations ...
```
这里`P`, `Q`, 和 `F`分别代表了估计误差协方差矩阵、过程噪声协方差矩阵以及状态转换模型矩阵。这些矩阵的具体数值取决于具体应用场景下的物理特性[^2]。
#### 编写预测函数
基于上述准备好的数据结构,现在编写用于执行预测步骤的功能模块:
```c
void predict(StateVector *state, double dt){
// Update state prediction using the motion model.
state->pos_x += state->vel_x * dt;
state->pos_y += state->vel_y * dt;
// Implementing the full Kalman filter equations would involve updating matrices like P with respect to time step 'dt'.
}
```
请注意,在实际应用中除了更新状态外还需按照标准公式调整协方差矩阵P以及其他相关项[^1]。
#### 测量更新部分
当接收到新的传感器读数时,应该调用另一个负责融合新旧信息并修正当前最佳猜测的方法:
```c
void update(StateVector *predicted_state, Measurement measurement){
// Calculate residual between predicted and actual measurements...
// Compute Kalman gain K based on current estimates of uncertainty...
// Apply correction term derived from weighted average of innovation/error terms.
// Finally adjust our belief about system's true condition by applying corrections computed above.
}
```
这段伪代码展示了典型的EKF(Extended Kalman Filter)中的校正流程的一部分操作。
以上就是针对五维情况的一个简化版框架描述;具体的数学细节和优化技巧则依赖于特定的任务需求和个人偏好而有所不同。值得注意的是,随着维度增加,计算复杂性和内存占用都会显著增长,因此实践中往往需要考虑性能因素做出适当折衷。
阅读全文
相关推荐








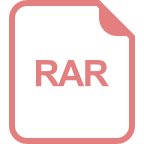








