两个服务之间的文件传递Java实现
时间: 2024-10-13 11:11:56 浏览: 19
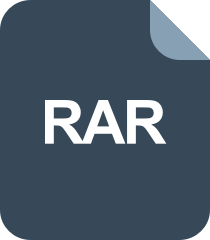
Java 两台服务器之间传递文件
在Java中,两个服务之间传递文件通常涉及到网络通信。常见的做法有通过HTTP、FTP、Socket等方式。这里我将以HTTP为例,展示一种简单的文件上传和下载方式:
1. **文件上传**:
使用`java.net.URLConnection`或第三方库如Apache HttpClient发送POST请求,包含文件内容。例如:
```java
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
public void uploadFile(String url, File file) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
MultipartEntityBuilder builder = MultipartEntityBuilder.create()
.addBinaryBody("file", new FileInputStream(file), ContentType.APPLICATION_OCTET_STREAM, file.getName());
HttpPost httpPost = new HttpPost(url);
httpPost.setEntity(builder.build());
HttpResponse response = httpClient.execute(httpPost);
// 处理响应...
}
```
2. **文件下载**:
则发送GET请求获取文件内容,并保存到本地。例如:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
public void downloadFile(String url, String outputPath) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet(url);
HttpResponse response = httpClient.execute(httpGet);
try (FileOutputStream fos = new FileOutputStream(outputPath)) {
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = response.getEntity().getContent().read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
}
// 关闭连接和实体...
}
```
阅读全文
相关推荐
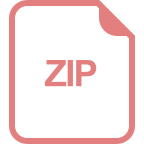
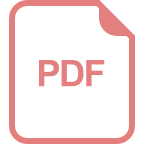
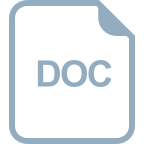
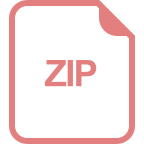
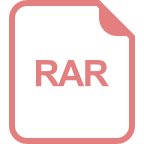
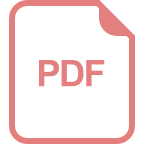
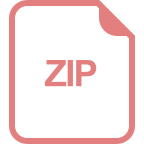
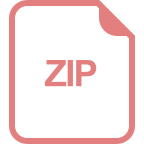
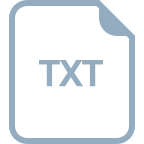
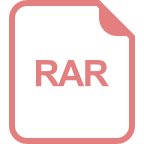
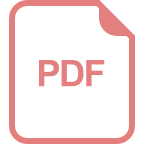
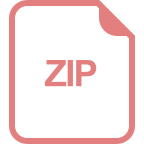
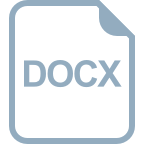
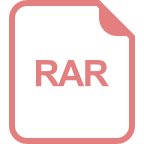
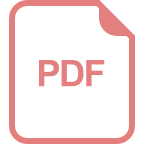
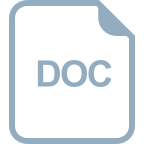
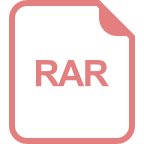
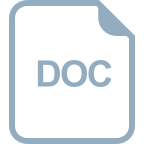