ransac算法阈值挑选
时间: 2023-09-11 09:03:20 浏览: 92
RANSAC算法中的阈值选择是一个重要的参数,它决定了哪些样本点被认为是“内点”(inliers)或“外点”(outliers)。阈值的选择需要根据具体的问题和数据集进行调整。
在RANSAC算法中,每次迭代时,随机选择一个最小子集样本来拟合模型,并将其他样本点与该模型进行比较。如果某个样本点与模型的拟合误差小于阈值,则认为该样本点是内点,否则是外点。
阈值的选择应该考虑以下几个因素:
1. 数据噪声水平:如果数据噪声较小,可以选择较小的阈值,以便更精确地筛选出内点。如果数据噪声较大,则需要选择较大的阈值,以允许更多的误差。
2. 模型复杂度:如果拟合的模型复杂度较高,例如高次多项式拟合,可能需要选择较大的阈值。因为复杂的模型更容易受到噪声的干扰,需要更大的容错能力。
3. 数据集大小:如果数据集较小,可以适当选择较小的阈值,以便更好地利用有限的数据。如果数据集较大,可以选择较大的阈值,以便更快地收敛。
总之,阈值的选择是一个经验性的过程,需要根据具体情况进行调整和优化。可以通过实验和验证来确定最佳的阈值,以获得满意的RANSAC拟合结果。
相关问题
RANSAC 算法的内点阈值
RANSAC 算法的内点阈值是指在进行模型拟合时,用于判断数据点是否属于内点的阈值。具体来说,对于给定的模型,RANSAC 算法计算每个数据点到该模型的距离,并将距离小于内点阈值的数据点归为内点,距离大于内点阈值的数据点归为外点。
内点阈值的选择取决于数据的特性以及模型的要求。一般来说,较小的内点阈值可以筛选出更精确的内点,但也可能将一些真正属于模型的外点误分类为内点。相反,较大的内点阈值可以容忍更多的噪声和异常点,但可能会导致估计出的模型不够精确。
因此,在选择内点阈值时需要权衡精度和鲁棒性。一种常见的做法是通过试验不同的内点阈值,并根据实际应用中的需求和评估结果来选择最佳的阈值。
需要注意的是,不同问题和数据集可能需要不同的内点阈值。例如,在拟合直线时,内点阈值可以表示为与直线距离的最大容忍值;在拟合平面时,内点阈值可以表示为与平面距离的最大容忍值。
希望这个解释对你有帮助!如果还有其他问题,请随时提问。
RANSAC算法中的阈值怎么设定
在RANSAC算法中,阈值的设定通常是依据具体问题而定的,它与输入数据的噪声水平有关。阈值设置得太小,可能会导致错误的数据点被纳入模型中,从而影响最终的结果;阈值设置得太大,可能会导致正确的数据点被排除在外,从而影响最终的结果。因此,需要根据具体问题来选择合适的阈值。一般来说,可以通过试验不同的阈值,然后选择能够得到最优模型的阈值。
相关推荐
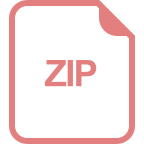












