写一个股票交易收益计算器
时间: 2024-09-15 12:13:50 浏览: 72
当你编写一个股票交易收益计算器时,你需要考虑几个关键因素:
1. **买入价格**:这是投资者最初购买股票的价格。
2. **卖出价格**:当股票被卖出时的价格,这可能高于或低于买入价。
3. **股数**:投资者持有的股票数量。
4. **交易费用**:买入和卖出股票通常会涉及到佣金或其他交易费用。
以下是一个简单的Python示例,用于计算单只股票的简单投资回报,不包括税费和其他复杂费用:
```python
class StockCalculator:
def __init__(self, buy_price, sell_price, shares):
self.buy_price = buy_price
self.sell_price = sell_price
self.shares = shares
def calculate_profit(self):
profit_per_share = self.sell_price - self.buy_price
total_profit = profit_per_share * self.shares
return total_profit
def calculate_total_cost(self):
return self.buy_price * self.shares
def net_profit(self):
total_cost = self.calculate_total_cost()
return self.calculate_profit() - total_cost
# 使用示例
buy_price = float(input("请输入买入价格: "))
sell_price = float(input("请输入卖出价格: "))
shares = int(input("请输入股数: "))
calculator = StockCalculator(buy_price, sell_price, shares)
profit = calculator.net_profit()
print(f"总利润: {profit} 元")
# 如果你想加入交易费用,你可以添加一个方法,比如:
def calculate_net_profit_with_commission(profit, commission_rate):
return profit - (profit * commission_rate)
commission_rate = float(input("请输入交易佣金率(如0.01表示1%): "))
net_profit = calculate_net_profit_with_commission(profit, commission_rate)
print(f"扣除佣金后的净利润: {net_profit} 元")
```
在这个例子中,`StockCalculator` 类包含了基本的利润计算方法。如果需要更复杂的逻辑,例如处理多个交易、复利、税金等,你可以扩展这个类。
阅读全文
相关推荐
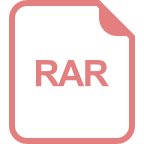
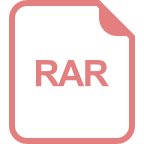
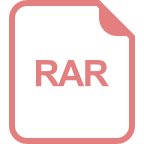


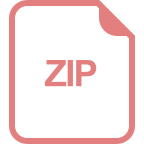
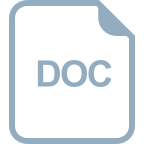
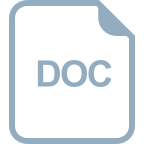



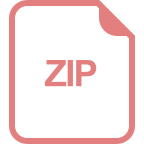
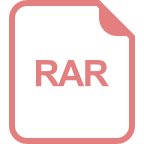
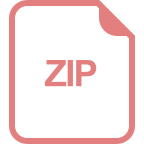
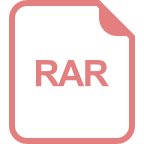
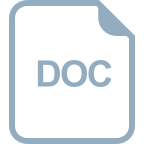

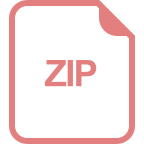