C++用单链表实现学生管理系统
时间: 2024-10-14 14:14:55 浏览: 41
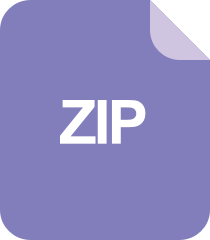
C和C++和单链表实现图书管理系统.zip
在C++中,我们可以使用单链表数据结构来实现一个简单的学生管理系统。首先,我们需要创建一些基本的数据结构,如`Student`节点,包含学生的姓名、学号等信息;然后创建一个`LinkedList`类来管理这些节点。
```cpp
// 学生节点定义
struct Student {
std::string name;
int id;
// 其他可能需要的属性...
// 构造函数和析构函数
Student(const std::string& name, int id) : name(name), id(id) {}
~Student() {}
};
// 单链表模板类
template <typename T>
class LinkedList {
private:
struct Node {
T data;
Node* next;
};
Node* head;
public:
LinkedList() : head(nullptr) {}
// 插入新学生
void addStudent(const T& student) {
Node* newNode = new Node{student, nullptr};
if (head == nullptr)
head = newNode;
else
Node* current = head;
while (current->next != nullptr)
current = current->next;
current->next = newNode;
}
// 删除学生
void removeStudent(int id) {
if (head == nullptr)
return;
if (head->data.id == id) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next != nullptr && current->next->data.id != id)
current = current->next;
if (current->next != nullptr)
current->next = current->next->next;
else
std::cout << "Student not found." << std::endl;
}
// 打印所有学生
void printStudents() {
Node* current = head;
while (current != nullptr) {
std::cout << "Name: " << current->data.name << ", ID: " << current->data.id << std::endl;
current = current->next;
}
}
};
// 使用示例
int main() {
LinkedList<Student> students;
students.addStudent(Student{"张三", 1001});
students.addStudent(Student{"李四", 1002});
students.printStudents();
students.removeStudent(1001);
return 0;
}
```
阅读全文
相关推荐
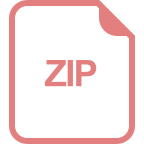
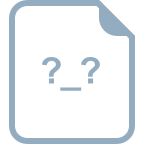
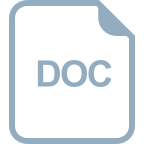


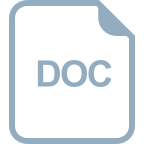
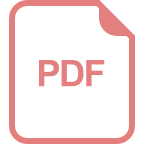
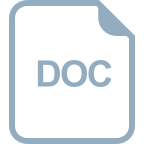
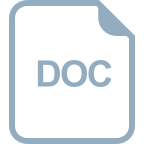
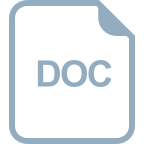

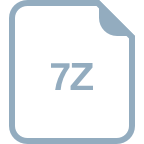
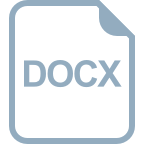
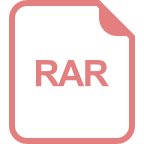
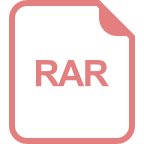
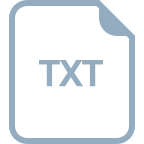
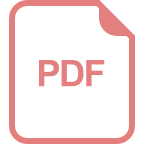