设计一个C++面向对象程序设计的简单几何图形题库系统代码
时间: 2024-10-18 11:14:37 浏览: 49
设计一个简单的C++面向对象程序设计的几何图形题库系统,我们可以创建一个名为`Geometry`的基类,然后为一些基本的几何形状(如点、线段、矩形、圆形等)创建派生类。这里是一个简化的例子:
```cpp
#include <iostream>
#include <string>
// 基类 Geometry
class Geometry {
protected:
std::string type;
public:
Geometry(const std::string& shapeType) : type(shapeType) {}
virtual void displayInfo() const = 0;
};
// 点 Point
class Point : public Geometry {
private:
double x, y;
public:
Point(double xCoord, double yCoord) : x(xCoord), y(yCoord), type("Point") {}
void displayInfo() const override {
std::cout << "Point at (" << x << ", " << y << ")" << std::endl;
}
};
// 线段 Segment
class Segment : public Geometry {
private:
Point start, end;
public:
Segment(Point startPt, Point endPt) : start(startPt), end(endPt), type("Segment") {}
void displayInfo() const override {
std::cout << "Segment from (" << start.x << ", " << start.y << ") to (" << end.x << ", " << end.y << ")" << std::endl;
}
};
// 简化版矩形和圆形,它们也有各自的属性和显示信息方法
class Rectangle : public Geometry {
private:
double width, height;
public:
// 类构造函数
Rectangle(double w, double h) : width(w), height(h), type("Rectangle") {}
void displayInfo() const override {
std::cout << "Rectangle with dimensions (" << width << ", " << height << ")" << std::endl;
}
};
class Circle : public Geometry {
private:
double radius;
public:
Circle(double r) : radius(r), type("Circle") {}
void displayInfo() const override {
std::cout << "Circle with radius " << radius << std::endl;
}
};
int main() {
Point p(1, 2);
Segment s(p, Point(4, 5));
Rectangle r(6, 7);
Circle c(8);
p.displayInfo();
s.displayInfo();
r.displayInfo();
c.displayInfo();
return 0;
}
```
在这个示例中,每个几何形状都有其特定的信息(坐标、尺寸或半径),并通过虚函数`displayInfo()`来展示。`main()`函数展示了如何创建并操作各种几何对象。
阅读全文
相关推荐
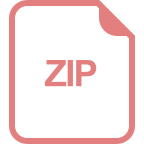
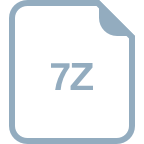
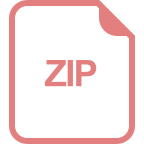















