操作系统实验-动态分区分配方式的模拟的全部代码
时间: 2024-12-12 18:20:15 浏览: 31
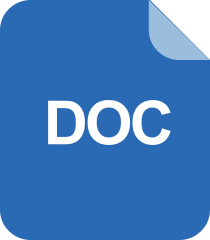
计算机操作系统实验-动态分区分配方式的模拟.doc

动态分区分配是一种内存管理策略,在操作系统实验中,我们通常使用链表来模拟动态创建、删除和分配大小不一的分区。以下是一个简单的C语言代码示例,展示了如何使用链表结构实现动态分区分配:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// 定义分区节点结构
typedef struct {
size_t size;
void* ptr; // 分区地址
bool is_free; // 是否为空闲
struct PartitionNode* next; // 链接下一个分区
} PartitionNode;
// 动态分区管理器
typedef struct {
PartitionNode* free_list; // 未分配的分区链表头部
size_t total_memory; // 总内存大小
} MemoryManager;
// 初始化内存管理器
MemoryManager* init_memory_manager(size_t total_size) {
MemoryManager* manager = malloc(sizeof(MemoryManager));
if (!manager) {
printf("Memory allocation failed.\n");
return NULL;
}
manager->free_list = NULL;
manager->total_memory = total_size;
return manager;
}
// 分配分区
void* allocate_partition(MemoryManager* mm, size_t size) {
if (size > mm->total_memory) {
printf("Cannot allocate a partition larger than the total memory.\n");
return NULL;
}
// 遍历空闲分区列表
for (PartitionNode* node = mm->free_list; node != NULL; node = node->next) {
if (node->is_free && node->size >= size) {
// 将分区从空闲列表移除并标记为已分配
node->is_free = false;
mm->free_list = node->next;
// 返回分区地址
return node->ptr;
}
}
// 如果没有足够大的空闲分区,增加一个新的分区
void* new_ptr = malloc(size);
if (!new_ptr) {
printf("Memory allocation for new partition failed.\n");
return NULL;
}
// 创建新的分区节点
PartitionNode* newNode = malloc(sizeof(PartitionNode));
newNode->size = size;
newNode->ptr = new_ptr;
newNode->is_free = false;
newNode->next = mm->free_list;
mm->free_list = newNode;
return new_ptr;
}
// 释放分区
void deallocate_partition(MemoryManager* mm, void* ptr) {
for (PartitionNode* node = mm->free_list; node != NULL; node = node->next) {
if (node->ptr == ptr) {
// 将分区添加回空闲列表
node->is_free = true;
mm->free_list = node;
break;
}
}
}
// 示例用法
int main() {
MemoryManager* manager = init_memory_manager(1000); // 假设总内存为1000字节
if (manager) {
void* allocated = allocate_partition(manager, 50); // 分配50字节
// 使用分配的内存
if (allocated) {
// ...其他操作...
deallocate_partition(manager, allocated); // 释放内存
} else {
printf("Failed to allocate memory.\n");
}
free(manager);
} else {
printf("Failed to initialize memory manager.\n");
}
return 0;
}
```
阅读全文
相关推荐
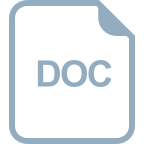
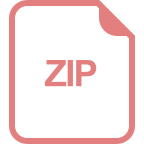

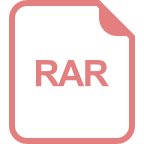
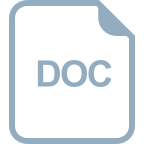
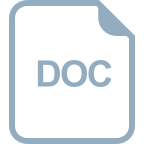
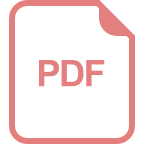
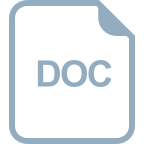
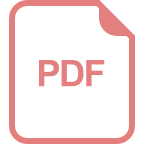
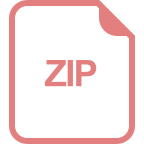
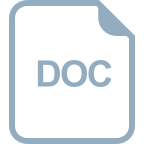
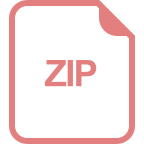
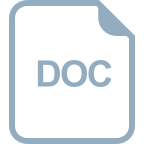
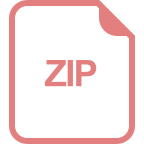
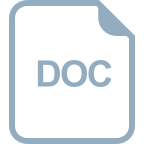
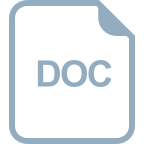
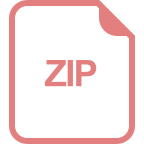
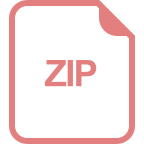