公交线路查询系统代码c++
时间: 2024-09-28 10:04:47 浏览: 21
公交线路查询系统是一个基于C++编写的程序,主要用于用户输入起点和终点,然后检索相关信息如路线、站点、公交车辆等。以下是实现这种系统的简化流程:
1. **数据结构**:
- 创建一个包含公交线路信息的数据结构,比如`LineInfo`,包含线路ID、起始站、终点站、路线列表等属性。
- 使用`vector`或`map`存储线路信息,便于快速查找。
2. **用户界面**:
- 设计一个命令行界面,接收用户的起点和终点输入。
- 可能需要提供菜单选项供用户选择查询方式(如按线路名、站点名搜索)。
3. **查询功能**:
- 根据用户输入,遍历存储的线路信息,匹配起始站和目的地,找到合适的线路。
- 提供结果输出,包括线路名称、经过的站点以及预计时间等。
4. **错误处理**:
- 检查用户输入的有效性,如输入的站点不存在等,并给出相应的提示。
5. **可能的扩展**:
- 考虑集成实时公交信息API,提供更准确的预测到达时间和路线变更通知。
```cpp
#include <iostream>
#include <string>
#include <vector>
// 示例数据结构
struct LineInfo {
std::string id;
std::string startStation;
std::string endStation;
std::vector<std::string> stations; // 线路经过的所有站点
};
class BusRouteQuery {
public:
void searchRoute(std::string start, std::string end) {
for (const auto& line : lines) {
if (line.startStation == start && line.endStation == end) {
printRoute(line);
return;
}
}
std::cout << "No route found from " << start << " to " << end << std::endl;
}
private:
std::vector<LineInfo> lines; // 存储的公交线路数据
void printRoute(const LineInfo& line) {
std::cout << "Route: " << line.id << ", Stations: ";
for (const auto& station : line.stations) {
std::cout << station << " -> ";
}
}
};
int main() {
BusRouteQuery querySystem;
// 初始化线路数据...
// 接收用户输入并调用searchRoute方法...
return 0;
}
```
相关推荐
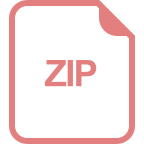
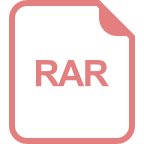
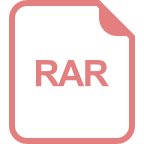

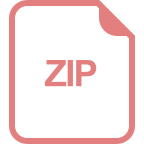
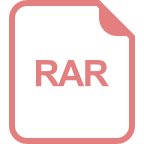
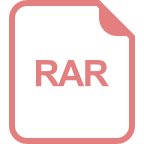
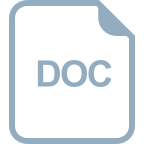
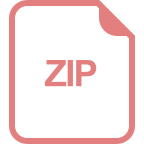
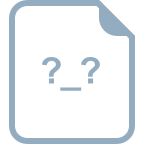
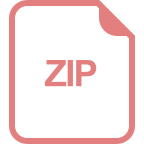
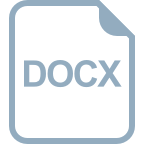
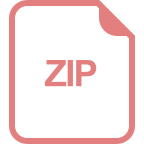
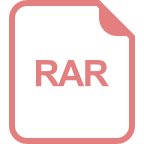
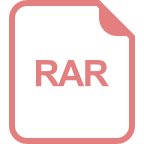
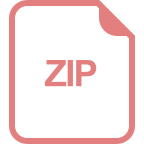
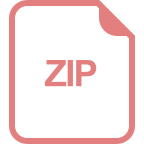
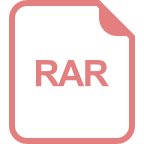