pstr_cpy将s2字符串的内容复制到s1字符串,返回新的s1的长度。 pstr_cat将s2字符串的内容连接到s1字符串的后面,返回新的s1的长度。 参数中,size是s1的数组长度
时间: 2024-04-12 11:32:35 浏览: 108
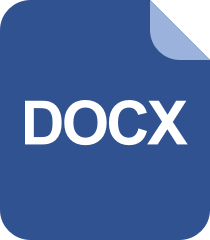
数据结构-c语言-带main函数-串2-串复制-根据标准库函数strcpy ()和定义求串复制函数的两种方法。
pstr_cpy函数的实现如下:
```c
#includestring.h>
int p_cpy(char *s1, const char *s2, size) {
int len = strlen(s2);
int copy_len = len < size ? len : size - 1;
strncpy(s1, s2, copy_len);
s1[copy_len] = '\0';
return copy_len;
}
```
pstr_cat函数的实现如下:
```c
#include <string.h>
int pstr_cat(char *s1, const char *s2, int size) {
int len1 = strlen(s1);
int len2 = strlen(s2);
int copy_len = len2 < size - len1 ? len2 : size - len1 - 1;
strncat(s1, s2, copy_len);
return len1 + copy_len;
}
```
这两个函数都使用了C标准库中的字符串函数`strncpy`和`strncat`来进行字符串的复制和连接。其中,`size`参数表示`s1`数组的长度,用于限制复制或连接的最大长度。函数返回的是新的`s1`字符串的长度(不包括结尾的空字符)。
阅读全文
相关推荐




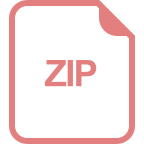
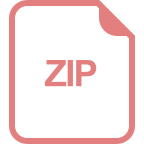
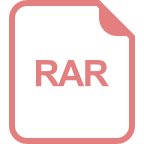
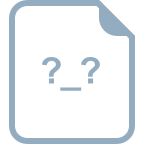





