python实现文本规范化
时间: 2024-09-10 18:23:32 浏览: 37
Python实现文本规范化可以通过以下步骤实现:
1. 文本清洗:去除HTML标签、特殊字符、数字等无用信息。
2. 文本切分:将文本切分为词。
3. 删除多余字符:删除停用词、标点符号等无用信息。
4. 词形还原:将单词还原为其原始形式。
5. 词性标注:标注每个单词的词性。
6. 去除低频词:去除出现频率较低的单词。
以下是一个简单的Python代码示例,实现了文本规范化的前三个步骤:
```
import nltk
import re
import string
# 文本清洗
def clean_text(text):
# 去除HTML标签
text = re.sub('<[^>]*>', '', text)
# 去除特殊字符
text = re.sub('[^a-zA-Z0-9\s]', '', text)
# 去除数字
text = re.sub('\d+', '', text)
# 去除多余空格
text = re.sub('\s+', ' ', text)
return text.strip()
# 文本切分
def tokenize_text(text):
sentences = nltk.sent_tokenize(text)
word_tokens = [nltk.word_tokenize(sentence) for sentence in sentences]
return word_tokens
# 删除多余字符
def remove_characters(tokens):
stop_words = nltk.corpus.stopwords.words('english')
pattern = re.compile('[{}]'.format(re.escape(string.punctuation)))
filtered_tokens = [pattern.sub('', token) for token in tokens if token not in stop_words]
return filtered_tokens
# 示例
sample_text = "The brown fox wasn't that quick and he couldn't win the ra"
cleaned_text = clean_text(sample_text)
tokens = tokenize_text(cleaned_text)
filtered_tokens = [remove_characters(sentence_tokens) for sentence_tokens in tokens]
print(filtered_tokens)
```
阅读全文
相关推荐
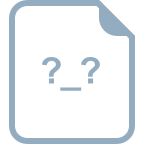
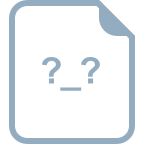
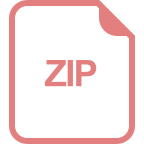
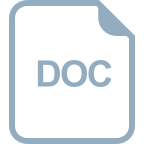
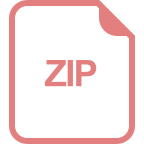
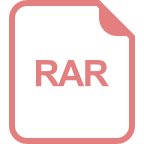
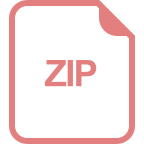
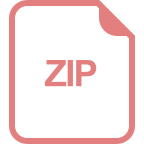
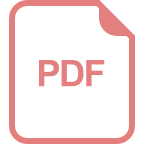
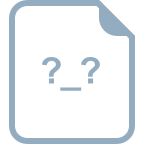
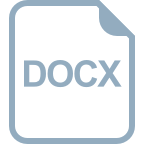
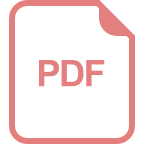
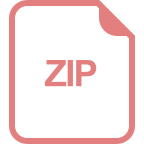
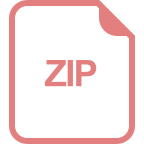
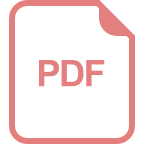
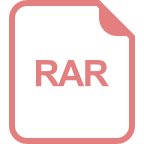
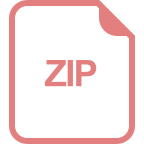
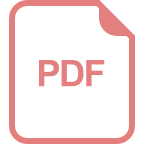
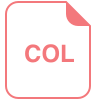
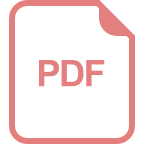