HBase Filter主要在Scan和Get过程中进行数据过滤,通过设置一些过滤条件来实现,如设置RowKey、列名或者列值的过滤条件。 根据这段话的描述,写一个java代码
时间: 2024-10-23 20:02:55 浏览: 31
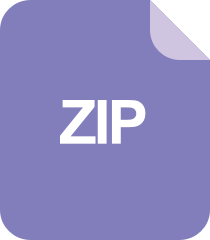
mumu-hbase项目是一个初步了解和学习hbase的demo项目,通过这个项目了解到hbase列数据库是由表、
在HBase中,`Filter`是用于扫描(Scan)和获取(Get)过程中的数据筛选。下面是一个简单的`FilterList`示例,它结合了多种过滤器,比如`RowFilter`(基于行键)、`ColumnPrefixFilter`(基于列前缀)以及`SingleColumnValueFilter`(基于单列值),用于从表中检索满足特定条件的数据:
```java
import org.apache.hadoop.hbase.Cell;
import org.apache.hadoop.hbase.CellScanner;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.HTableInterface;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.client.Get;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.filter.*;
import org.apache.hadoop.hbase.util.Bytes;
public class HBaseFilterExample {
public static void main(String[] args) throws Exception {
Configuration config = HBaseConfiguration.create();
TableName tableName = TableName.valueOf("your_table_name");
// 创建连接
Connection connection = ConnectionFactory.createConnection(config);
try (Admin admin = connection.getAdmin()) {
// 检查表是否存在并创建,如果不存在
if (!admin.tableExists(tableName)) {
// 创建表
byte[][] families = {Bytes.toBytes("family1"), Bytes.toBytes("family2")};
admin.createTable(TableName.of(tableName), families);
}
// 定义过滤器列表
FilterList filterList = new FilterList(FilterList.Operator.MUST_PASS_ALL);
filterList.addFilter(new RowFilter(CompareOperator.EQUAL, Bytes.toBytes("target_row_key"))); // 行键过滤
filterList.addFilter(new ColumnPrefixFilter(Bytes.toBytes("prefix_column"))); // 列名前缀过滤
filterList.addFilter(new SingleColumnValueFilter(Bytes.toBytes("family1"), Bytes.toBytes("columnQualifier"),
CompareOp.EQUAL, Bytes.toBytes("expected_value"))); // 单列值过滤
// 执行get操作,并应用过滤器
Get get = new Get(Bytes.toBytes("query_row_key")); // 查询行键
Result result = hTable.get(get, filterList);
// 遍历结果并打印符合条件的Cell
CellScanner scanner = result.cellScanner();
while (scanner.advance()) {
Cell cell = scanner.current();
System.out.printf("Family: %s, Qualifier: %s, Value: %s%n", cell.getFamilyArray(), cell.getQualifierArray(), cell.getValue());
}
} finally {
connection.close();
}
}
private static HTableInterface hTable;
// 在main方法之前初始化hTable,这里省略
}
```
在这个例子中,你需要将`"your_table_name"`、`"target_row_key"`、`"prefix_column"`、`"expected_value"`以及`"query_row_key"`替换为实际的值。
阅读全文
相关推荐
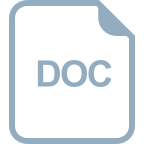
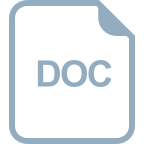

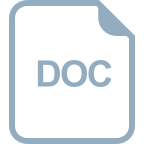
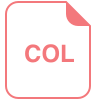
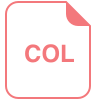
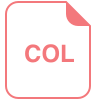
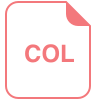
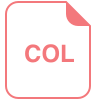
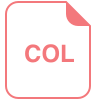
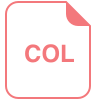
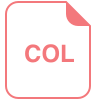
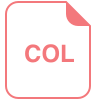
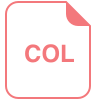
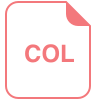


