c#设计一个实现记事本的min程序
时间: 2024-11-09 21:26:10 浏览: 21
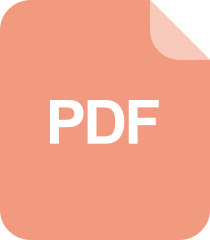
C#实现简单记事本程序
在C#中设计一个简单的文本编辑器(类似记事本),你可以创建一个控制台应用程序,并利用基本的文本输入和输出功能来实现。以下是简要步骤:
1. **创建项目**: 打开Visual Studio或其他支持C#的IDE,新建一个控制台应用项目。
2. **命名空间与类**: 定义一个名为`Notepad`的类,它将是记事本的核心。你可以将其放在`ConsoleApp1`或自定义命名空间里。
```csharp
using System;
using System.IO;
namespace ConsoleApp1
{
public class Notepad
{
// 程序逻辑将在这里编写
}
}
```
3. **添加成员**: 添加一些基本的属性和方法,如用于打开、读取、编辑和保存文件的函数。
```csharp
public class Notepad
{
private string filePath;
public Notepad(string fileName)
{
filePath = fileName;
}
public void OpenFile()
{
if (File.Exists(filePath))
{
Console.WriteLine("Opening file...");
ReadContent();
}
else
{
Console.WriteLine("File not found.");
}
}
private void ReadContent()
{
try
{
string content = File.ReadAllText(filePath);
Console.WriteLine(content);
}
catch (Exception ex)
{
Console.WriteLine($"Error reading file: {ex.Message}");
}
}
public void EditContent(string newContent)
{
try
{
File.WriteAllText(filePath, newContent);
Console.WriteLine("Content updated.");
}
catch (Exception ex)
{
Console.WriteLine($"Error editing file: {ex.Message}");
}
}
public void Save()
{
if (string.IsNullOrEmpty(newContent))
{
Console.WriteLine("No changes to save.");
}
else
{
File.WriteAllText(filePath, newContent);
Console.WriteLine("Changes saved.");
}
}
}
```
4. **主程序**: 创建`Main`方法来实例化并交互使用记事本类。
```csharp
class Program
{
static void Main(string[] args)
{
Notepad notePad = new Notepad("example.txt");
Console.WriteLine("Welcome to simple Notepad!");
while (true)
{
Console.WriteLine("\nChoose an action:");
Console.WriteLine("1. Open");
Console.WriteLine("2. Edit");
Console.WriteLine("3. Save");
Console.WriteLine("4. Exit");
int choice = Convert.ToInt32(Console.ReadLine());
switch (choice)
{
case 1:
notePad.OpenFile();
break;
case 2:
Console.Write("Enter new content: ");
string newContent = Console.ReadLine();
notePad.EditContent(newContent);
break;
case 3:
notePad.Save();
break;
case 4:
Console.WriteLine("Exiting the program...");
return;
default:
Console.WriteLine("Invalid choice. Please try again.");
break;
}
}
}
}
```
阅读全文
相关推荐
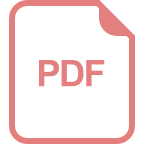
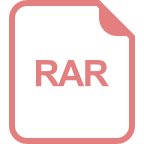
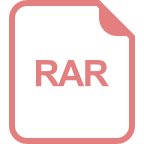
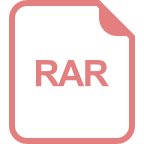
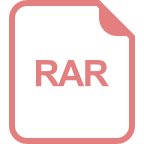
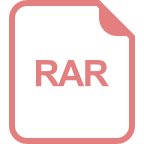
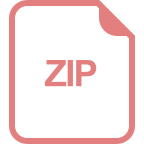
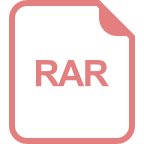
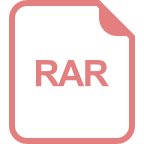
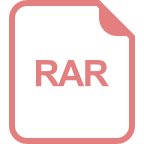
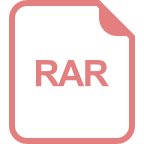
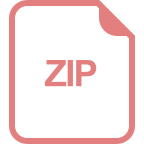
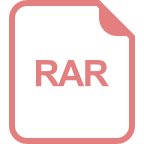
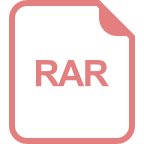
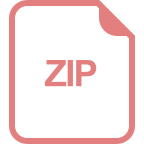
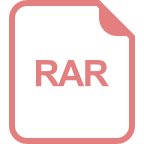
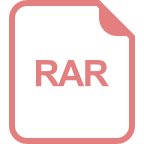