编写一个程序,模拟剪刀石头布游戏,用户出拳后,程序随机生成剪刀、石头、布, 并输出游戏结果
时间: 2024-09-29 12:02:10 浏览: 35
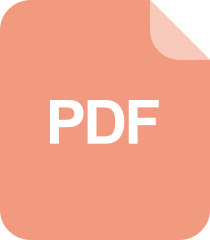
js实现石头剪刀布游戏
这是一个简单的Python程序,可以模拟剪刀石头布的游戏过程:
```python
import random
def game():
choices = ["石头", "剪刀", "布"]
user_input = input("请出拳(石头、剪刀、布):")
if user_input not in choices:
print("无效的选择,请重新输入!")
return game()
computer_choice = random.choice(choices)
result = {
"石头": {"石头": "平局", "剪刀": "赢", "布": "输"},
"剪刀": {"石头": "输", "剪刀": "平局", "布": "赢"},
"布": {"石头": "赢", "剪刀": "输", "布": "平局"}
}
print(f"你出了{user_input},电脑出了{computer_choice},结果是:{result[user_input][computer_choice]}")
game()
```
这个程序会循环询问用户选择,直到输入有效选项为止。然后,它会随机选择电脑的选项,并根据规则判断胜负。
阅读全文
相关推荐
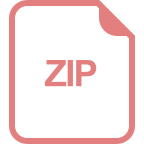
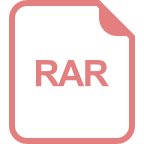













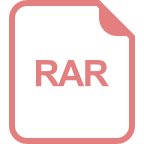
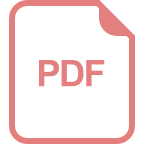
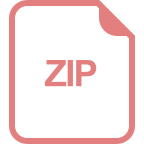