用python写自动关机程序怎么做
时间: 2024-09-22 19:06:18 浏览: 59
在Python中编写一个自动关机程序非常简单,你可以使用`subprocess`模块来执行操作系统级别的命令。下面是一个简单的例子,它会在指定秒数后自动关闭电脑:
```python
import time
import subprocess
def shutdown_after(seconds):
print(f"将在{seconds}秒后关闭电脑")
time.sleep(seconds) # 等待指定秒数
command = "shutdown /s /t 0" # Windows系统的关机命令,/t 0表示立即执行
try:
subprocess.call(command, shell=True)
except Exception as e:
print("自动关机失败:", str(e))
# 调用函数,比如你想5分钟后关机,可以这么用:
shutdown_after(60*5) # 参数是秒数,这里是300秒(5分钟)
```
这个脚本假设你的系统是Windows。如果是其他系统如macOS,可以使用`os.system`替代`subprocess.call`,命令变为`os.system('sudo shutdown -h now')`。
请注意,在实际应用中,需要谨慎使用这样的功能,因为它可能会无意间导致电脑突然关闭,影响正在进行的工作。
相关问题
用python做一个关机代码
以下是一个简单的Python关机代码:
```python
import os
# 输入关机时间(单位为秒)
shutdown_time = int(input("请输入关机时间(单位为秒):"))
# 执行关机命令
os.system(f"shutdown -s -t {shutdown_time}")
```
运行上述代码后,程序会要求你输入关机时间,单位为秒。程序会自动计算出关机时间,并执行关机命令。请注意,这个命令会强制关机,所有未保存的文件都将丢失。因此,在使用这个代码前,请确保你已经保存好了所有的工作。如果需要取消关机,请使用 `shutdown -a` 命令。
用python给我做一个工具包包括小闹钟,计算器,自动关机,记事本,网站打开器,快捷键盘等
好的,以下是一个基于Python的工具包,其中包括小闹钟、计算器、自动关机、记事本、网站打开器、快捷键盘等功能。
```python
import time
import webbrowser
import os
from tkinter import *
from tkinter import messagebox
# 小闹钟
def alarm():
alarm_time = entry1.get()
while True:
time.sleep(1)
current_time = time.strftime("%H:%M:%S")
if current_time == alarm_time:
messagebox.showinfo("Alarm", "Time's up!")
break
# 计算器
def calculate():
expression = entry2.get()
try:
result = eval(expression)
messagebox.showinfo("Result", f"The result is {result}")
except:
messagebox.showerror("Error", "Invalid expression")
# 自动关机
def shutdown():
time = int(entry3.get())
os.system(f"shutdown /s /t {time}")
# 记事本
def notepad():
os.system("notepad")
# 网站打开器
def open_website():
website = entry5.get()
webbrowser.open(website)
# 快捷键盘
def shortcut():
root2 = Toplevel()
root2.title("Shortcuts")
root2.geometry("300x200")
label = Label(root2, text="Press Ctrl + C to copy")
label.pack(pady=10)
def copy():
root.clipboard_clear()
root.clipboard_append(entry6.get())
root.bind("<Control-c>", lambda x: copy())
# 创建GUI
root = Tk()
root.title("Toolbox")
root.geometry("400x500")
# 小闹钟
label1 = Label(root, text="Alarm Clock")
label1.pack(pady=10)
entry1 = Entry(root, width=10, font=("Helvetica", 20))
entry1.pack(pady=10)
button1 = Button(root, text="Set Alarm", command=alarm)
button1.pack(pady=10)
# 计算器
label2 = Label(root, text="Calculator")
label2.pack(pady=10)
entry2 = Entry(root, width=30, font=("Helvetica", 20))
entry2.pack(pady=10)
button2 = Button(root, text="Calculate", command=calculate)
button2.pack(pady=10)
# 自动关机
label3 = Label(root, text="Shutdown Timer (in secs)")
label3.pack(pady=10)
entry3 = Entry(root, width=10, font=("Helvetica", 20))
entry3.pack(pady=10)
button3 = Button(root, text="Shutdown", command=shutdown)
button3.pack(pady=10)
# 记事本
label4 = Label(root, text="Notepad")
label4.pack(pady=10)
button4 = Button(root, text="Open Notepad", command=notepad)
button4.pack(pady=10)
# 网站打开器
label5 = Label(root, text="Website Opener")
label5.pack(pady=10)
entry5 = Entry(root, width=30, font=("Helvetica", 20))
entry5.pack(pady=10)
button5 = Button(root, text="Open Website", command=open_website)
button5.pack(pady=10)
# 快捷键盘
label6 = Label(root, text="Shortcut Keyboard")
label6.pack(pady=10)
entry6 = Entry(root, width=30, font=("Helvetica", 20))
entry6.pack(pady=10)
button6 = Button(root, text="Open Shortcuts", command=shortcut)
button6.pack(pady=10)
root.mainloop()
```
你可以在控制台中运行这个程序,或者将代码保存到.py文件中,双击运行。
阅读全文
相关推荐






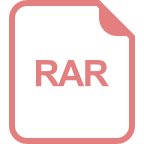








