c语言更改目录下所有文件权限
时间: 2023-07-16 10:02:35 浏览: 239
### 回答1:
在C语言中,我们可以使用系统调用函数`chmod()`来更改文件权限。更改目录下所有文件的权限的基本步骤如下:
1. 使用`opendir()`函数打开指定目录,并将返回的目录指针保存到一个变量中。
2. 使用`readdir()`函数来读取目录中的文件。
3. 对于每个读取到的文件,使用`stat()`函数获取文件的信息,包括文件名和权限。
4. 使用`chmod()`函数将文件的权限更改为目标权限。
5. 重复步骤3和4,直到所有文件都被处理完。
6. 使用`closedir()`函数关闭目录。
下面是一个简单的示例程序,用于更改目录下所有文件的权限为目标权限:
```c
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <dirent.h>
#include <unistd.h>
void changePermissions(const char *dirPath, mode_t targetMode) {
DIR *dir = opendir(dirPath);
if (!dir) {
perror("opendir");
return;
}
struct dirent *entry;
char filePath[256];
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_REG) {
snprintf(filePath, sizeof(filePath), "%s/%s", dirPath, entry->d_name);
struct stat fileStat;
if (stat(filePath, &fileStat) == -1) {
perror("stat");
continue;
}
mode_t oldMode = fileStat.st_mode;
mode_t newMode = (oldMode & ~07777) | targetMode;
if (chmod(filePath, newMode) == -1) {
perror("chmod");
continue;
}
}
}
closedir(dir);
}
int main() {
const char *dirPath = "/path/to/directory";
mode_t targetMode = S_IRUSR | S_IWUSR | S_IRGRP | S_IWGRP | S_IROTH | S_IWOTH;
changePermissions(dirPath, targetMode);
return 0;
}
```
以上示例代码为更改目录下所有普通文件的权限为读写权限,包括所有用户。你可以根据需求修改目录路径和目标权限。
### 回答2:
要使用C语言更改目录下所有文件的权限,首先需要使用`opendir()`函数打开目录,然后使用`readdir()`函数读取目录中的文件。接下来,可以使用`stat()`函数获取文件的权限信息,包括所有者、组和其他用户的权限。使用`chmod()`函数可以更改文件的权限。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <sys/stat.h>
int main() {
DIR *dir;
struct dirent *entry;
struct stat fileinfo;
// 打开目录
dir = opendir("目录路径");
if (dir == NULL) {
printf("无法打开目录\n");
exit(1);
}
// 读取目录中的文件
while ((entry = readdir(dir)) != NULL) {
// 跳过 . 和 .. 目录
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0)
continue;
// 构建文件路径
char filepath[256];
snprintf(filepath, sizeof(filepath), "%s/%s", "目录路径", entry->d_name);
// 获取文件的权限信息
if (stat(filepath, &fileinfo) < 0) {
printf("无法获取文件信息:%s\n", filepath);
continue;
}
// 更改文件的权限为 777
if (chmod(filepath, S_IRWXU | S_IRWXG | S_IRWXO) < 0) {
printf("无法更改文件权限:%s\n", filepath);
continue;
}
printf("已更改文件权限:%s\n", filepath);
}
// 关闭目录
closedir(dir);
return 0;
}
```
以上示例代码演示了如何使用C语言更改目录下所有文件的权限为777。请将代码中的"目录路径"替换为实际的目录路径。该代码使用`stat()`函数获取文件信息,使用`chmod()`函数更改文件权限。需要注意的是,这段代码只能在拥有足够权限的情况下运行,否则可能会导致权限错误。
### 回答3:
要想使用C语言更改目录下所有文件的权限,需要使用系统函数和目录遍历技术。
首先,我们需要使用<sys/stat.h>头文件中的chmod()函数来更改文件的权限。该函数的原型如下:
int chmod(const char *path, mode_t mode);
其中,path是文件的路径名,mode是权限模式。通过调用该函数,我们可以更改文件的权限。
然后,我们需要使用<dirent.h>头文件中的opendir()和readdir()函数来遍历目录下的所有文件。这两个函数的原型如下:
DIR *opendir(const char *name);
struct dirent *readdir(DIR *dir);
其中,opendir()函数用于打开一个目录,返回一个指向目录流的指针,readdir()函数用于读取目录中的文件,返回一个指向dir的下一个目录项的指针。
接下来,我们可以使用递归的方式逐层遍历目录下的所有文件夹和文件。对于文件夹,我们需要调用chmod()函数将其权限更改为所需的权限。对于文件,我们可以直接调用chmod()函数更改其权限。
最后,为了避免出现权限更改失败或者无权限访问的问题,我们需要在代码中进行错误处理和权限检查。
总结起来,使用C语言更改目录下所有文件的权限,需要使用系统函数和目录遍历技术,具体操作步骤如下:打开目录,遍历目录中的文件,对文件夹进行递归遍历,对文件进行权限更改。
阅读全文
相关推荐


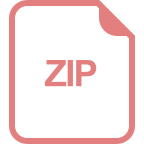
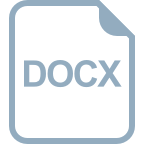
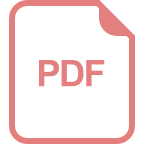
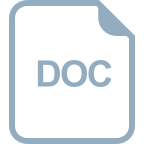
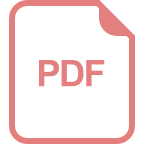
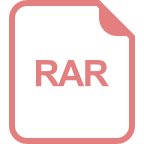
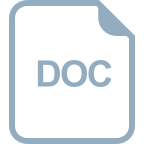
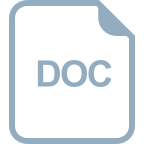





