$fetch post params
时间: 2023-11-24 18:08:00 浏览: 204
以下是使用fetch进行POST请求并传递参数的示例代码:
```javascript
fetch('接口', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: new URLSearchParams({
'参数1': '值1',
'参数2': '值2'
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
```
其中,'接口'是你要请求的URL地址,'参数1'和'参数2'是你要传递的参数名,'值1'和'值2'是对应的参数值。在headers中,'Content-Type'指定了请求体的数据格式为'application/x-www-form-urlencoded',这是一种常用的格式,用于序列化字符串。在body中,使用了URLSearchParams()来操作参数,将参数名和参数值以键值对的形式传递进去。
需要注意的是,fetch返回的是一个Promise对象,需要使用then()方法来处理响应结果。在then()方法中,可以使用response.json()将响应体解析为JSON格式的数据,然后再进行处理。如果请求出现错误,可以使用catch()方法来捕获错误并进行处理。
相关问题
前端post请求同时传递params和body
前端发送POST请求通常需要携带两个部分的数据:params(查询参数)和body(请求体)。在HTTP协议中,params通常用于GET请求,而POST请求则用于提交更复杂的数据,比如表单数据、JSON对象等。
在JavaScript(如使用fetch API或axios库)中,你可以这样做:
1. **使用`URLSearchParams` for params**:
```javascript
const url = 'https://example.com/api';
const params = new URLSearchParams();
params.set('key', 'value');
let bodyParams = params.toString(); // 对于GET请求
// 对于POST请求,可以单独处理params
fetch(url + '?' + bodyParams, {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: JSON.stringify({ key: 'value' }) // 同时包含body
})
```
2. **直接拼接到body for POST请求** (JSON格式):
```javascript
fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
paramsKey: 'value', // 查询参数
requestBody: { ... }, // 请求体内容
}),
})
```
或者,如果你使用axios库:
```javascript
axios.post(url, {
params: { key: 'value' },
data: { requestBody: { ... } }, // 请求体
})
```
记住,在发送JSON body时,你需要设置正确的Content-Type头,通常是`'application/json'`。
我想用js文件发送一个带headers和params的post请求
A:
你可以使用Fetch API或XMLHttpRequest对象发送带headers和params的post请求,以下是使用Fetch API的示例代码:
```js
const headers = new Headers({
'Content-Type': 'application/json',
'Authorization': 'Bearer <token>'
});
const params = {
username: 'john',
email: 'john@example.com'
};
const fetchOptions = {
method: 'POST',
headers: headers,
body: JSON.stringify(params)
};
fetch('https://example.com/api/user', fetchOptions)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
```
在上面的代码中,我们使用Headers对象来设置请求头部,JSON.stringify()方法将params对象转换为JSON字符串,fetch()函数发送POST请求,并在响应中使用json()方法解析JSON响应。你可以根据你的实际情况更改这些参数。
如果你想使用XMLHttpRequest对象发送post请求,你可以这样编写:
```js
const xhr = new XMLHttpRequest();
const endpoint = 'https://example.com/api/user';
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE) {
if (xhr.status === 200) {
console.log(xhr.responseText);
} else {
console.error(xhr.statusText);
}
}
};
xhr.open('POST', endpoint);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.setRequestHeader('Authorization', 'Bearer <token>');
const params = {
username: 'john',
email: 'john@example.com'
};
xhr.send(JSON.stringify(params));
```
在这个例子中,我们使用XMLHttpRequest对象来发送POST请求,并在响应中解析响应文本。我们还设置了请求头部,通过setRequestHeader()方法进行设置。
无论你选择哪种方式,都可以通过相应的参数来发送自定义头和参数的POST请求。
阅读全文
相关推荐















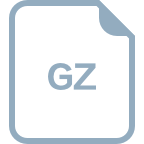