本题要求编写程序,将给定字符串去掉重复的字符后,按照字符ASCII码顺序从小到大排序后输出。 输入格式: 输入是一个以回车结束的非空字符串(少于80个字符)。 输出格式: 输出去重排序后的结果字符串。
时间: 2024-10-19 21:12:26 浏览: 17
要实现这个功能,你可以先创建一个函数来删除重复的字符,然后对剩余的字符进行ASCII码排序。以下是一个简单的C语言程序,使用`#include <stdio.h>`、`#include <ctype.h>` 和 `#include <string.h>` 来完成这个任务:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
// 函数用于去除字符串中的重复字符并保持ASCII顺序
void removeDuplicatesAndSort(char* str) {
int len = strlen(str);
if (len == 0) return; // 如果字符串为空,直接返回
// 创建一个新的字符串,用于存储不重复的字符
char result[90]; // 假设最多有89个不同的字符(包括空格)
memset(result, 0, sizeof(result));
// 遍历原字符串
for (int i = 0; i < len; i++) {
char c = tolower(str[i]); // 转换为小写,便于比较
if (result[c] == '\0') { // 如果新字符串中该字符位置是空,则添加
result[c] = c;
}
}
// 将结果字符串转换回小写并输出
for (int i = 0; result[i] != '\0'; i++) {
printf("%c", tolower(result[i])); // 输出字符
}
}
int main() {
char input[81];
fgets(input, sizeof(input), stdin); // 从标准输入读取字符串,包含回车符
// 移除行尾的换行符
input[strcspn(input, "\n")] = '\0';
removeDuplicatesAndSort(input);
return 0;
}
```
在这个程序中,我们首先将所有字符转换为小写字母以便进行大小写的忽略。接着遍历原字符串,如果新字符串中对应的ASCII位置是空(`\0`),则添加当前字符。最后,将处理后的字符串逐个输出。
阅读全文
相关推荐
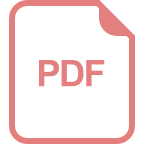
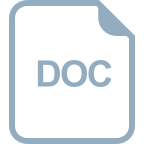
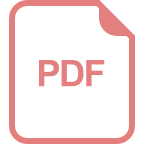














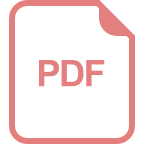
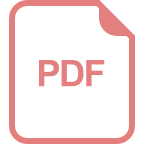
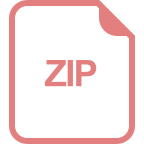