JAVA两个时间戳获取间隔时间
时间: 2024-10-20 21:10:01 浏览: 27
在Java中,你可以通过`java.time`包中的`Duration`类来计算两个时间戳之间的间隔时间。假设你有两个`long`类型的表示时间的毫秒值,例如`long timestamp1`和`long timestamp2`,你可以这样做:
```java
import java.time.Duration;
import java.time.Instant;
public long getTimeDifference(long timestamp1, long timestamp2) {
Instant instant1 = Instant.ofEpochMilli(timestamp1);
Instant instant2 = Instant.ofEpochMilli(timestamp2);
Duration duration = Duration.between(instant1, instant2);
return duration.toMillis(); // 返回以毫秒为单位的时间差
}
```
这个函数首先将时间戳转换成`Instant`对象,然后利用`Duration.between()`方法计算两个时刻之间的持续时间,最后返回间隔的毫秒数。
相关问题
java比较2个时间戳间隔天数
在Java中,可以使用 `java.time.Instant` 类来比较两个时间戳之间的天数间隔。以下是一个示例代码:
```java
import java.time.Instant;
import java.time.temporal.ChronoUnit;
public class TimestampIntervalExample {
public static void main(String[] args) {
long timestamp1 = 1628025600; // 2021-08-04T00:00:00Z
long timestamp2 = 1628112000; // 2021-08-05T00:00:00Z
Instant instant1 = Instant.ofEpochSecond(timestamp1);
Instant instant2 = Instant.ofEpochSecond(timestamp2);
long daysBetween = ChronoUnit.DAYS.between(instant1, instant2);
System.out.println("Days between " + instant1 + " and " + instant2 + " is: " + daysBetween);
}
}
```
在此示例中,我们使用 `Instant` 类来表示时间戳。我们使用 `Instant.ofEpochSecond` 方法将时间戳转换为 `Instant` 对象。然后,我们使用 `ChronoUnit.DAYS.between` 方法计算两个时间戳之间的天数间隔。
此代码将输出以下内容:
```
Days between 2021-08-04T00:00:00Z and 2021-08-05T00:00:00Z is: 1
```
请注意,使用 `Instant` 类时要注意时区问题。如果您需要在不同时区之间进行比较,请使用 `java.time.OffsetDateTime` 或 `java.time.ZonedDateTime` 类。
java生成固定时间内的时间戳
在Java中,如果你需要生成特定时间范围内的固定时间戳,你可以使用`java.time`包下的`LocalDateTime`或`Instant`类配合`Duration`类来实现。以下是一个示例:
```java
import java.time.Duration;
import java.time.Instant;
public class Main {
public static void main(String[] args) {
// 设定起始时间和结束时间(这里假设开始时间是当前时间,结束时间是5分钟后)
LocalDateTime startTime = LocalDateTime.now();
LocalDateTime endTime = startTime.plusMinutes(5);
// 获取两个时间点之间的跨度,即固定时间内的时间间隔
Duration duration = Duration.between(startTime, endTime);
// 如果你想获取每个时间段的开始时间戳,可以这样做:
Instant startTimestamp = startTime.atZone(ZoneId.systemDefault()).toInstant();
Instant endTimestamp = endTime.atZone(ZoneId.systemDefault()).toInstant();
System.out.println("Start timestamp: " + startTimestamp);
System.out.println("End timestamp: " + endTimestamp);
}
}
```
在这个例子中,`startTimestamp`和`endTimestamp`分别表示了固定时间范围内的起始和结束时间戳。
阅读全文
相关推荐
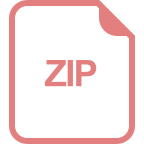
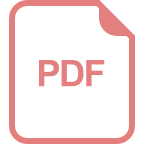
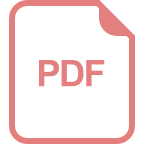
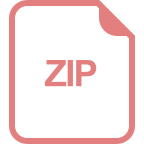
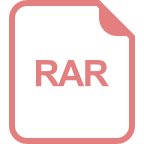
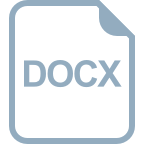
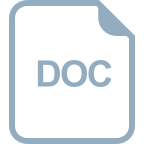
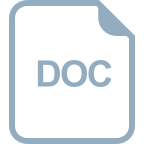








