如何在c++添加图片
时间: 2024-09-09 17:00:43 浏览: 36
在C++中添加图片通常涉及到图形用户界面(GUI)的编程。由于C++标准库本身并不支持图形操作,你需要使用第三方图形库,如Qt、wxWidgets、SFML、SDL或者Allegro等来实现这一功能。以下是使用其中一些流行库的简要介绍:
1. Qt:这是一个跨平台的C++库,用于开发图形用户界面应用程序以及非GUI程序,比如命令行工具和服务器。Qt支持通过其QPixmap或QImage类来加载和显示图片。
示例代码:
```cpp
#include <QPixmap>
#include <QGraphicsView>
#include <QGraphicsScene>
QPixmap pixmap("path/to/image.png");
QGraphicsScene scene;
scene.addPixmap(pixmap);
QGraphicsView view(&scene);
view.show();
```
2. wxWidgets:这是一个开源的跨平台GUI库,它允许你在Windows、Mac OS X、Linux等操作系统上用C++编写图形用户界面。
示例代码:
```cpp
#include <wx/wx.h>
#include <wx/image.h>
class MyApp : public wxApp
{
public:
virtual bool OnInit();
};
IMPLEMENT_APP(MyApp)
bool MyApp::OnInit()
{
wxInitAllImageHandlers();
wxImage::AddHandler(new wxPNGHandler());
wxFrame *frame = new wxFrame(NULL, wxID_ANY, "Image Example");
wxImage image("path/to/image.png");
wxStaticBitmap *bitmap = new wxStaticBitmap(frame, wxID_ANY, wxBitmap(image));
frame->SetSize(400, 400);
frame->Centre();
frame->Show(true);
return true;
}
```
3. SDL(Simple DirectMedia Layer):这主要用于游戏和多媒体应用的开发,它提供了一系列低层次的访问音频、键盘、鼠标、游戏手柄以及图形硬件等功能。
示例代码:
```cpp
#include <SDL.h>
#include <SDL_image.h> // 需要这个扩展库来加载图片
int main(int argc, char* argv[])
{
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("SDL Image Example", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 640, 480, 0);
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED);
SDL_Surface* imgSurface = IMG_Load("path/to/image.png");
SDL_Texture* imgTexture = SDL_CreateTextureFromSurface(renderer, imgSurface);
SDL_FreeSurface(imgSurface);
SDL_RenderClear(renderer);
SDL_RenderCopy(renderer, imgTexture, NULL, NULL);
SDL_RenderPresent(renderer);
SDL_Delay(5000); // 等待5秒
SDL_DestroyTexture(imgTexture);
SDL_DestroyRenderer(renderer);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
```
在选择库时,请根据你的具体需求(如平台兼容性、项目类型和性能要求等)进行选择。
阅读全文
相关推荐
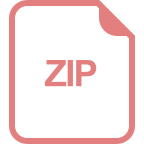
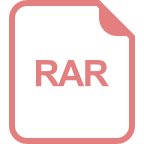
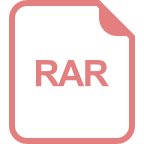
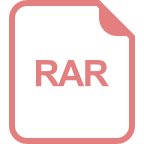
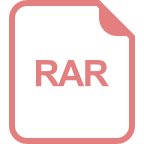





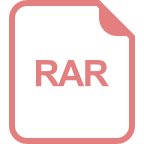
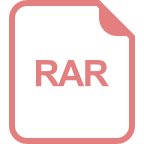
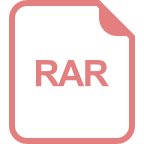
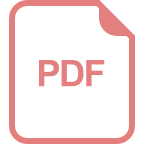
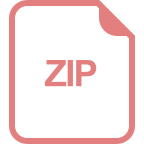
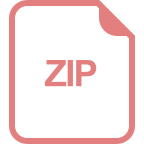


