用python写贪吃蛇小游戏的系统测试
时间: 2024-12-06 14:34:01 浏览: 16
在Python编写贪吃蛇游戏的系统测试时,我们关注的是游戏的基本功能是否正常工作,包括但不限于以下几个方面:
1. **初始化测试**:检查游戏是否能正确启动,蛇头、食物的位置是否随机生成,并且初始长度和速度设置是否合理。
```python
def test_initialization():
game = SnakeGame()
assert game.is_running is True
assert len(game.snake) == game.LENGTH
```
2. **移动测试**:验证蛇能否按照用户的输入方向移动,边界条件如碰壁、吃到食物和长大的处理是否正确。
```python
def test_movement():
game = SnakeGame()
game.move(Direction.UP)
assert game.snake[0][1] > game.snake[1][1]
# ...其他方向的测试
game.eat_food()
assert len(game.snake) == game.LENGTH + 1
```
3. **碰撞检测测试**:确认蛇头是否可以穿透墙壁,以及是否能正确处理蛇体与其他部分的碰撞。
```python
def test_collision_detection():
game = SnakeGame()
game.snake[0][0] = 10, 10 # 将蛇头放在边界上
game.move(Direction.DOWN)
assert not game.is_running
```
4. **循环测试**:长时间运行游戏看看是否有性能问题,比如游戏是否卡顿,内存占用是否稳定等。
```python
def test_game_loop_stability():
import time
game = SnakeGame()
for _ in range(100):
game.update()
time.sleep(0.1) # 模拟游戏循环
assert game.alive
```
5. **异常处理测试**:确保当游戏遇到错误情况,如非法输入或系统崩溃时,能够有适当的反馈或恢复机制。
```python
def test_error_handling():
try:
game = SnakeGame(move='invalid_direction')
except ValueError:
assert True # 预期会抛出异常
```
阅读全文
相关推荐
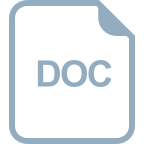
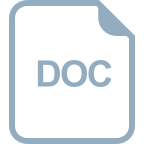
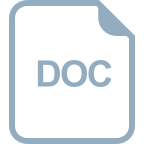
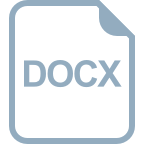
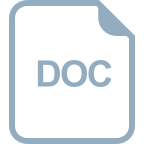
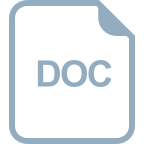
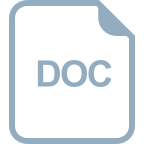
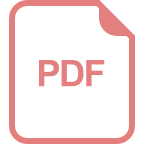
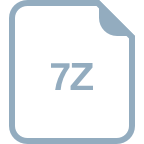
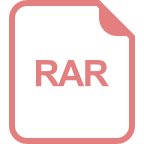
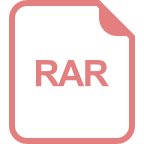
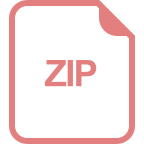
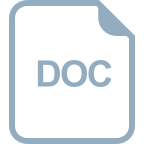
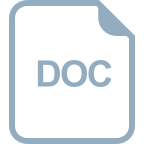
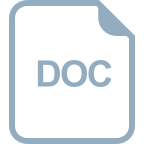
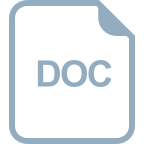
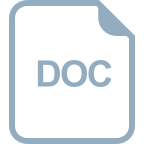
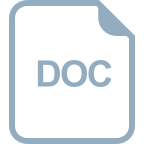
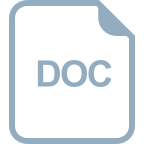