(1)请设计一个程序演示死锁避免算法(银行家算法)。要求该演示程序能显示各进程申请和释放资源以及系统动态分配资源的过程,便于用户观察和分析;如果系统不能分配,也应给出“系统进入不安全状态”的提示。进程和各类共享资源数量、已分配量、申请量最好随机给出。
时间: 2024-12-01 19:19:28 浏览: 5
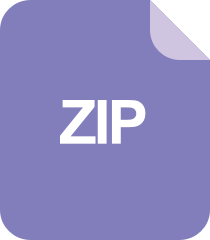
操作系统银行家算法避免死锁
为了演示死锁避免算法(例如银行家算法),我们可以编写一个简单的Python程序。首先,我们需要定义进程、资源池和银行家类。下面是一个简化版的示例,其中包含进程和资源的状态以及银行家决策过程:
```python
import random
import threading
class Resource:
def __init__(self, name, max_amount, allocated=0):
self.name = name
self.max_amount = max_amount
self.allocated = allocated
class Process:
def __init__(self, id, reqs):
self.id = id
self.requests = reqs
self.resources = {}
def acquire_resource(self, resource, amount):
if resource.allocated + amount <= resource.max_amount:
resource.allocated += amount
self.resources[resource.name] = amount
print(f"Process {self.id} acquired {amount} of {resource.name}")
else:
print(f"Process {self.id} cannot acquire more {resource.name}")
def release_resource(self, resource_name):
resource = self.resources.get(resource_name)
if resource is not None:
resource.allocated -= resource
if resource == 0:
del self.resources[resource_name]
print(f"Process {self.id} released {resource} of {resource_name}")
else:
print(f"Process {self.id} tried to release non-existent resource {resource_name}")
class Banker:
def __init__(self, resources):
self.resources = resources
self.safety_matrix = [[r.max_amount for r in resources] for _ in resources]
def can_allocate(self, process, current_allocations):
matrix = self.update_safety_matrix(current_allocations)
return self.is_safe(process.requests, matrix)
def update_safety_matrix(self, current_allocations):
# 根据当前分配更新矩阵
updated_matrix = []
for i, proc in enumerate(self.resources):
new_row = [max(0, self.safety_matrix[i][j] - current_allocations[j]) for j in range(len(self.resources))]
updated_matrix.append(new_row)
return updated_matrix
def is_safe(self, requests, matrix):
deadlock_found = False
for i, row in enumerate(matrix):
if any(row[j] < requests[j] for j in range(len(requests))):
deadlock_found = True
break
return not deadlock_found
def run_simulation(num_processes, resources, banker):
while True:
process = next((p for p in processes if p.requests), None) # 获取下一个请求资源的进程
if process:
allocations = [banker.can_allocate(process, current_allocations) for current_allocations in process.resource_allocations()]
if any(allocations):
selected_process, selected_allocation = max(zip(processes, allocations), key=lambda x: x[1])
selected_process.acquire_resource(selected_process.resources.keys()[selected_allocation], 1)
selected_process.resource_allocations.append(selected_allocation)
else:
print("System enters unsafe state")
break
else:
break
resources = [Resource('R1', 5), Resource('R2', 4)]
processes = [Process(i, random.randint(1, min(r.max_amount, len(resources))) for i in range(5)]
banker = Banker(resources)
threads = [threading.Thread(target=run_simulation, args=(len(processes), resources, banker)) for _ in processes]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
这个程序会随机生成进程对资源的需求,并模拟它们尝试获取资源的过程。银行家会根据资源池和当前分配情况判断是否允许分配。当系统进入不安全状态时,会打印出相应消息。你可以运行这个程序并在终端查看输出,以便观察和分析资源分配的情况。
阅读全文
相关推荐
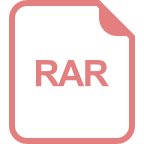
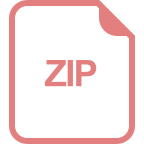
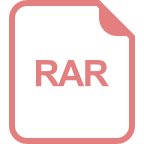
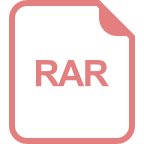
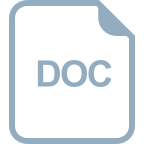
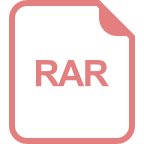
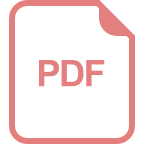
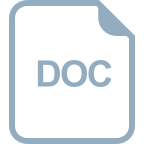
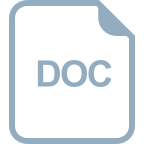
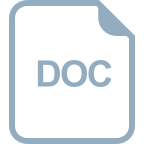
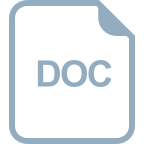
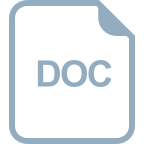
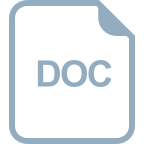
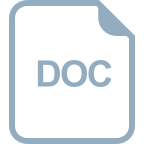
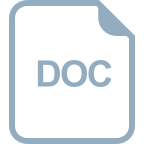
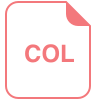

