使用 Python 实现简单的线性回归算
时间: 2024-11-18 16:19:23 浏览: 4
在Python中,我们可以使用内置的`numpy`和`matplotlib`库来实现简单的线性回归。这里有一个基本的例子,我们将使用Scikit-learn库,它提供了更方便的工具。
首先,你需要安装必要的库,如果尚未安装,可以使用pip命令:
```bash
pip install numpy matplotlib scikit-learn
```
然后,你可以按照以下步骤创建一个简单的线性回归模型:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
# 创建一些模拟数据
x = np.array([1, 2, 3, 4, 5]).reshape(-1, 1) # 输入特征
y = np.array([2, 4, 5, 4, 5]) # 目标值
# 创建并训练模型
model = LinearRegression()
model.fit(x, y)
# 预测新数据
new_x = np.array([[6], [7]])
predictions = model.predict(new_x)
# 绘制数据和拟合直线
plt.scatter(x, y, color='blue')
plt.plot(new_x, predictions, color='red', label="Linear Regression Line")
plt.xlabel("Input feature")
plt.ylabel("Target value")
plt.legend()
plt.show()
# 打印模型的相关参数
print("Intercept:", model.intercept_)
print("Slope:", model.coef_[0])
```
这个例子中,我们创建了一个线性模型,通过输入特征(这里是数字1到5)预测目标值。`fit()`函数训练模型,`predict()`函数用于做出新的预测。最后,我们用散点图展示了原始数据,并显示了模型预测的新点以及拟合的直线。
阅读全文
相关推荐
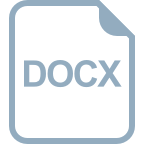
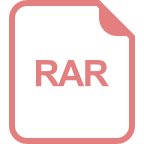
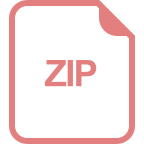
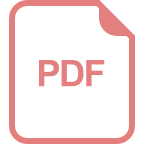
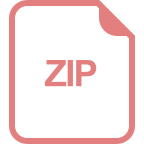
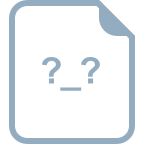
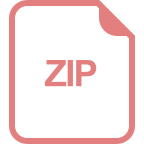
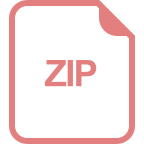
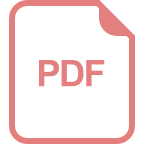
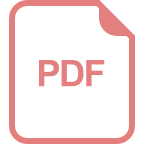
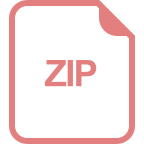
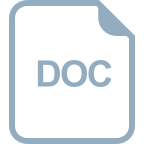
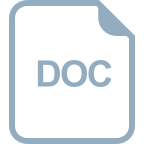
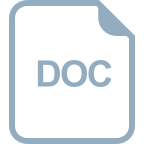
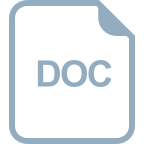
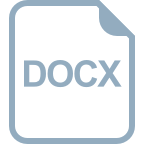