python生日快乐爱心烟花特效
时间: 2024-12-26 21:26:31 浏览: 4
### Python 实现生日快乐爱心烟花特效
为了实现一个带有“生日快乐”的爱心烟花特效,可以结合`pygame`和`turtle`这两个图形库的功能。下面提供一段综合了两者特性的代码示例。
#### 使用 `pygame` 创建窗口并显示文字
首先安装必要的库:
```bash
pip install pygame
```
接着编写如下Python脚本:
```python
import sys
import pygame
from pygame.locals import *
import math
import random
import turtle as t
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Happy Birthday with Heart Fireworks")
font = pygame.font.Font(None, 74)
def draw_text(text):
text_surface = font.render(text, True, (255, 255, 255))
screen.blit(text_surface, dest=(150, 250))
class Particle:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
angle = random.uniform(0, 2 * math.pi)
speed = random.uniform(3, 7)
self.dx = speed * math.cos(angle)
self.dy = -speed * math.sin(angle)
def move(self):
self.x += self.dx
self.y += self.dy + 0.1 # 加速度模拟重力
self.dx *= 0.98 # 减速因子模仿空气阻力
self.dy *= 0.98
def is_alive(self):
return abs(self.dx) > 0.1 or abs(self.dy) > 0.1
particles = []
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
screen.fill((0, 0, 0)) # 清屏操作
draw_text("HAPPY BIRTHDAY!")
if len(particles) < 100 and random.random() < 0.05:
particles.append(Particle(random.randint(200, 600), 500,
('red' if random.choice([True, False]) else 'pink')))
new_particles = []
for p in particles:
p.move()
if p.is_alive():
new_particles.append(p)
pygame.draw.circle(screen, p.color, (int(p.x), int(p.y)), 3)
particles = new_particles
pygame.display.flip()
pygame.time.delay(30)
# 添加Turtle绘制的心形图案作为静态装饰
t.hideturtle()
t.speed('fastest')
t.penup()
t.goto(-100, -100)
t.pendown()
def heart(k):
return 15*math.sin(k)**3
for i in range(400):
k = i*0.01
x = int(heart(k)*12)-100
y = -(k-3)*(k-3)+100
r, g, b = (random.randint(0, 255),
random.randint(0, 255),
random.randint(0, 255))
t.pencolor(r, g, b)
t.goto(x,y)
pygame.display.update()
```
此段程序利用了`pygame`来处理主要的游戏循环以及粒子系统的逻辑,并通过`turtle`模块在屏幕上画出了彩色的心形轨迹[^1]。注意这里简化了一些细节以便更好地理解整体结构;实际应用时可以根据需求调整参数使效果更加逼真。
阅读全文
相关推荐
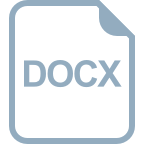
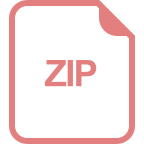















