java 根据Excel模板导出excel 写一个完整的代码
时间: 2024-10-10 11:02:43 浏览: 36
在Java中,你可以使用Apache POI库来处理Excel文件,包括读取模板和生成新的工作簿。下面是一个基本的示例,展示了如何根据Excel模板导出数据。请注意,这个例子假设你已经有了一个模板文件和预定义的数据结构。
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelExporter {
private static final String TEMPLATE_FILE_PATH = "path_to_your_template.xlsx";
private static final String OUTPUT_FILE_PATH = "path_to_output.xlsx";
public static void main(String[] args) throws IOException {
// 加载模板
FileInputStream inputStream = new FileInputStream(TEMPLATE_FILE_PATH);
Workbook workbookTemplate = new XSSFWorkbook(inputStream);
// 创建新的工作簿
Workbook workbookOutput = new XSSFWorkbook();
// 获取模板的工作表
Sheet sheetTemplate = workbookTemplate.getSheetAt(0);
// 创建新的工作表并获取引用
Sheet sheetOutput = workbookOutput.createSheet();
Row rowOutput = sheetOutput.createRow(0); // 新的一行
// 假设模板的第一行是标题,从第二行开始获取数据
int rowIndex = 1;
while (rowIndex < sheetTemplate.getLastRowNum()) {
Row templateRow = sheetTemplate.getRow(rowIndex++);
// 复制模板行到新工作表
copyRow(templateRow, rowOutput);
// 添加更多的行... (这里省略)
}
// 关闭流
inputStream.close();
// 将新工作簿写入文件
try (FileOutputStream outputStream = new FileOutputStream(OUTPUT_FILE_PATH)) {
workbookOutput.write(outputStream);
}
// 释放资源
workbookOutput.close();
}
private static void copyRow(Row sourceRow, Row destinationRow) {
for (Cell cell : sourceRow.getCells()) {
Cell destinationCell = destinationRow.createCell(cell.getColumnIndex());
switch (cell.getCellType()) { // 处理不同类型的数据
case STRING:
destinationCell.setCellValue(cell.getStringCellValue());
break;
case NUMERIC:
destinationCell.setCellValue(cell.getNumericCellValue());
break;
case BOOLEAN:
destinationCell.setCellValue(cell.getBooleanCellValue());
break;
default:
destinationCell.setCellValue(cell.getStringCellValue()); // 默认字符串处理
}
}
}
}
```
阅读全文
相关推荐
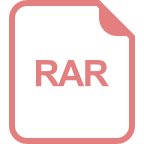
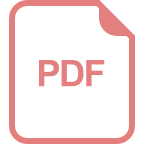
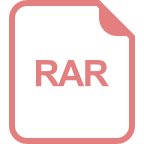
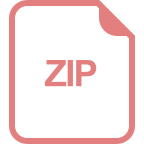
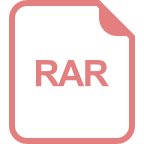
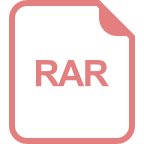
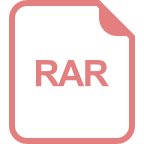
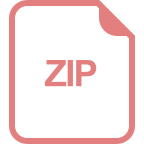
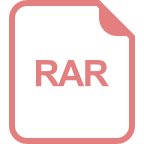







