C#控制台实现读写excel
时间: 2023-08-21 19:06:55 浏览: 101
要在C#控制台中实现读写Excel,可以使用Microsoft.Office.Interop.Excel库。首先,您需要添加对该库的引用。然后,您可以使用以下代码示例来实现读取和写入Excel文件。
读取Excel文件的示例代码如下:
```csharp
using Excel = Microsoft.Office.Interop.Excel;
using System.IO;
void ReadExcel(string filePath)
{
Excel.Application app = new Excel.Application();
Excel.Workbook workbook = app.Workbooks.Open(filePath);
Excel.Worksheet worksheet = workbook.Sheets\[1\];
int rowCount = worksheet.UsedRange.Rows.Count;
int columnCount = worksheet.UsedRange.Columns.Count;
for (int i = 1; i <= rowCount; i++)
{
for (int j = 1; j <= columnCount; j++)
{
string cellValue = worksheet.Cells\[i, j\].Value.ToString();
Console.Write(cellValue + " ");
}
Console.WriteLine();
}
workbook.Close();
app.Quit();
}
string filePath = Path.Combine(System.Environment.CurrentDirectory, "test.xlsx");
ReadExcel(filePath);
```
写入Excel文件的示例代码如下:
```csharp
using Excel = Microsoft.Office.Interop.Excel;
using System.IO;
void WriteExcel(double\[,\] data, string filePath)
{
Excel.Application app = new Excel.Application();
Excel.Workbook workbook = app.Workbooks.Add();
Excel.Worksheet worksheet = workbook.Sheets\[1\];
int rowCount = data.GetLength(0);
int columnCount = data.GetLength(1);
for (int i = 0; i < rowCount; i++)
{
for (int j = 0; j < columnCount; j++)
{
worksheet.Cells\[i + 1, j + 1\] = data\[i, j\].ToString();
}
}
workbook.SaveAs(filePath);
workbook.Close();
app.Quit();
}
double\[,\] data = new double\[5, 5\];
string filePath = Path.Combine(System.Environment.CurrentDirectory, "test.xlsx");
WriteExcel(data, filePath);
Console.WriteLine($"写入到{filePath}");
```
请注意,这些示例代码假设您已经安装了Microsoft Office并且在项目中添加了对Interop.Excel库的引用。此外,您还需要根据实际情况更改文件路径和数据。
#### 引用[.reference_title]
- *1* *2* *3* [C#读写Excel](https://blog.csdn.net/m0_37816922/article/details/128043199)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
阅读全文
相关推荐






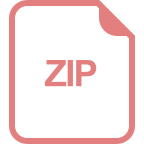

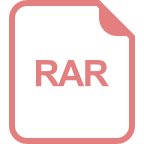


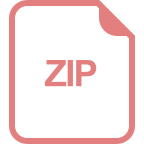



