String text = "**微信公众号:经年相伴**";
时间: 2024-08-16 16:07:54 浏览: 61
在这个Java字符串`text`中,你可以使用正则表达式或者特定的方法来提取"微信公众号:"后面的内容。这里以正则表达式为例:
```java
String text = "**微信公众号:经年相伴**";
Pattern pattern = Pattern.compile("(?<=微信公众号:).*"); // 匹配"微信公众号:"之后直到字符串结束的部分
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
String extractedText = matcher.group(); // 提取到的内容
System.out.println("提取的内容: " + extractedText); // 输出:“经年相伴”
}
```
这段代码创建了一个正则表达式模式,用于查找"微信公众号:"后面的一切字符(`.+`),并使用`Matcher`对象找到并提取匹配的文本。
相关问题
springboot整合微信公众号
Spring Boot 可以很方便地与微信公众号进行整合。下面是一个 Spring Boot 整合微信公众号的简单步骤:
1.创建一个微信公众号并获取 AppID 和 AppSecret。
2.在 pom.xml 中添加以下依赖:
```
<dependency>
<groupId>com.github.sd4324530</groupId>
<artifactId>weixin-java-tools-spring-boot-starter</artifactId>
<version>2.9.0</version>
</dependency>
```
3.在 application.properties 中配置 AppID 和 AppSecret:
```
wechat.appId=yourAppId
wechat.appSecret=yourAppSecret
wechat.token=yourToken
wechat.aesKey=yourAesKey
```
4.创建一个类来配置微信公众号:
```
@Configuration
public class WechatConfig {
@Autowired
private WechatProperties wechatProperties;
@Bean
public WxMpConfigStorage wxMpConfigStorage(){
WxMpInMemoryConfigStorage configStorage = new WxMpInMemoryConfigStorage();
configStorage.setAppId(wechatProperties.getAppId());
configStorage.setSecret(wechatProperties.getAppSecret());
configStorage.setToken(wechatProperties.getToken());
configStorage.setAesKey(wechatProperties.getAesKey());
return configStorage;
}
@Bean
public WxMpService wxMpService(WxMpConfigStorage wxMpConfigStorage){
WxMpService wxMpService = new WxMpServiceImpl();
wxMpService.setWxMpConfigStorage(wxMpConfigStorage);
return wxMpService;
}
}
```
5.在 Controller 中处理微信公众号的消息:
```
@RestController
@RequestMapping("/wechat")
public class WechatController {
@Autowired
private WxMpService wxMpService;
@GetMapping(produces = "text/plain;charset=utf-8")
public String authGet(@RequestParam(name = "signature", required = false) String signature,
@RequestParam(name = "timestamp", required = false) String timestamp,
@RequestParam(name = "nonce", required = false) String nonce,
@RequestParam(name = "echostr", required = false) String echostr) {
if (!wxMpService.checkSignature(timestamp, nonce, signature)) {
return "非法请求";
}
return echostr;
}
@PostMapping(produces = "application/xml; charset=UTF-8")
public String post(@RequestBody String requestBody,
@RequestParam("signature") String signature,
@RequestParam("timestamp") String timestamp,
@RequestParam("nonce") String nonce,
@RequestParam("openid") String openid,
@RequestParam(name = "encrypt_type", required = false) String encType,
@RequestParam(name = "msg_signature", required = false) String msgSignature) {
if (!wxMpService.checkSignature(timestamp, nonce, signature)) {
throw new IllegalArgumentException("非法请求,可能属于伪造的请求!");
}
WxMpXmlMessage wxMessage;
if (encType == null) {
wxMessage = WxMpXmlMessage.fromXml(requestBody);
} else {
wxMessage = WxMpXmlMessage.fromEncryptedXml(requestBody, wxMpService.getWxMpConfigStorage(), timestamp, nonce, msgSignature);
}
String content = "收到消息内容:" + wxMessage.getContent();
WxMpXmlOutMessage outMessage = WxMpXmlOutMessage.TEXT().content(content).fromUser(wxMessage.getToUser())
.toUser(wxMessage.getFromUser()).build();
return outMessage.toXml();
}
}
```
现在你就可以通过该 Controller 处理微信公众号的消息了。
java 微信公众号签名
生成微信公众号签名的过程需要经过以下几个步骤:
1. 将请求参数按照字典序排序。
2. 将排序后的参数列表组装成一个字符串,格式为 key1=value1&key2=value2&...。
3. 将参数字符串末尾加上微信公众号的 AppSecret。
4. 对加上 AppSecret 的字符串进行 SHA1 加密。
5. 将加密后的字符串转换成小写字母,即为微信公众号签名。
以下是一个 Java 实现的示例代码:
```java
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class WeChatSignatureGenerator {
private static final String ALGORITHM = "SHA1";
public static String generateSignature(String token, String timestamp, String nonce) {
List<String> params = new ArrayList<>();
params.add(token);
params.add(timestamp);
params.add(nonce);
Collections.sort(params);
String paramString = String.join("", params);
try {
MessageDigest md = MessageDigest.getInstance(ALGORITHM);
byte[] digest = md.digest(paramString.getBytes());
StringBuilder sb = new StringBuilder();
for (byte b : digest) {
sb.append(String.format("%02x", b));
}
return sb.toString();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
return null;
}
}
}
```
其中,`token` 是微信公众号的开发者 token,`timestamp` 和 `nonce` 是微信服务器发来的请求参数。调用 `generateSignature` 方法即可生成微信公众号签名。
阅读全文
相关推荐
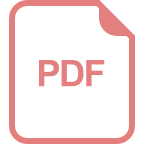
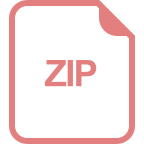
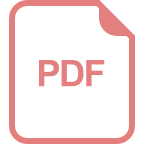
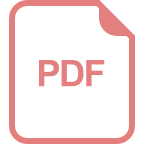
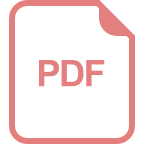
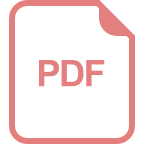
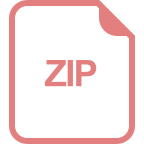
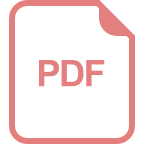
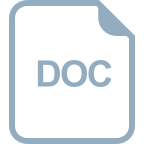
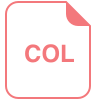
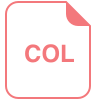
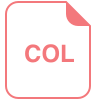
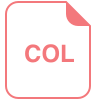
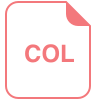
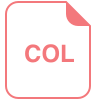

