用PYQT5实现数据标注
时间: 2024-09-12 15:11:57 浏览: 108
在PyQt5中实现数据标注通常涉及到创建用户界面(UI)以便用户可以交互地标记图像、文本或其他数据。以下是基本步骤:
1. **安装所需库**:首先确保已经安装了PyQt5和相关的数据处理库,如PIL (Python Imaging Library) 或 OpenCV用于图像操作。
```bash
pip install PyQt5 pillow opencv-python
```
2. **导入模块**:在Python脚本中,导入所需的PyQt5组件以及数据处理模块。
```python
import sys
from PyQt5.QtWidgets import QApplication, QLabel, QWidget, QVBoxLayout, QPushButton, QFileDialog
from PyQt5.QtGui import QPixmap, QImage
import cv2
```
3. **设计UI**:创建窗口,并添加标签(QLabel)显示图片,按钮(BUTTON)用来选择文件和保存标注结果。可以使用`QVBoxLayout`布局管理器来组织控件。
```python
class AnnotationApp(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
# 创建窗口
self.setWindowTitle('Image Annotation')
self.setGeometry(100, 100, 800, 600)
# 添加布局
layout = QVBoxLayout()
self.setLayout(layout)
# 图片展示区域
self.image_label = QLabel()
layout.addWidget(self.image_label)
# 文件选择按钮
select_button = QPushButton('Select Image')
select_button.clicked.connect(self.open_image)
layout.addWidget(select_button)
# 保存标注按钮
save_button = QPushButton('Save Annotation')
save_button.setEnabled(False)
layout.addWidget(save_button)
self.show()
def open_image(self):
file_path = QFileDialog.getOpenFileName(self, 'Open Image', '', "Images (*.png *.jpg)")
if file_path[0]:
image = cv2.imread(file_path[0])
pixmap = QPixmap.fromImage(QImage(image.data, image.shape[1], image.shape[0], QImage.Format_RGB888))
self.image_label.setPixmap(pixmap)
save_button.setEnabled(True)
```
4. **实现标注功能**:这通常需要额外的事件处理,比如鼠标点击事件来绘制矩形框或添加注释,然后将标注信息保存到文件。这部分可能会涉及一些图形学的知识和自定义函数。
5. **保存标注结果**:当用户完成标注后,你可以通过读取`pixmap`或者其他方式获取修改过的图像数据,并将其保存到文件。
阅读全文
相关推荐
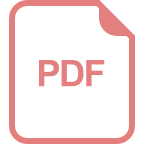
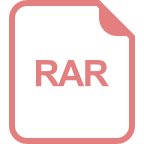
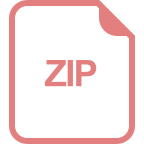
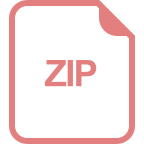
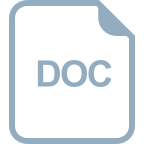
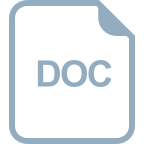
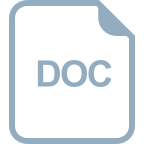

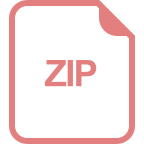
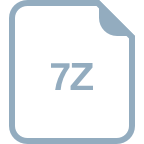
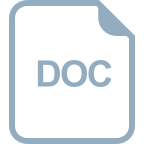
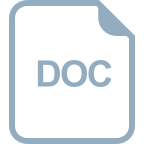
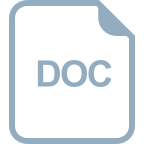
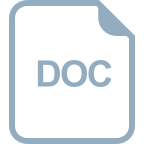
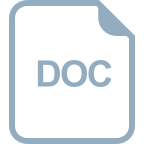
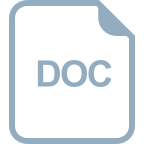

