java 上传图片到华为云oss
时间: 2024-06-07 09:02:06 浏览: 124
以下是使用jeecg-boot框架将图片上传到华为云OBS的步骤:
1.在华为云OBS中创建一个存储桶,并获取Access Key ID和Secret Access Key。
2.在jeecg-boot项目中添加OBS SDK依赖,可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.obs</groupId>
<artifactId>obs-sdk-java</artifactId>
<version>3.20.3</version>
</dependency>
```
3.在jeecg-boot项目中添加OBS配置信息,可以在application.yml文件中添加以下配置:
```yaml
obs:
endpoint: https://your-endpoint.obs.cn-north-1.myhuaweicloud.com
access-key: your-access-key-id
secret-key: your-secret-access-key
bucket-name: your-bucket-name
```
4.修改upload方法,添加上传到OBS的逻辑:
```java
public static String upload(MultipartFile file, String bizPath, String uploadType) {
String url = "";
try {
if (CommonConstant.UPLOAD_TYPE_MINIO.equals(uploadType)) {
url = MinioUtil.upload(file, bizPath);
} else if (CommonConstant.UPLOAD_TYPE_OSS.equals(uploadType)) {
url = OssBootUtil.upload(file, bizPath);
} else if (CommonConstant.UPLOAD_TYPE_OBS.equals(uploadType)) {
url = ObsBootUtil.upload(file, bizPath);
}
} catch (Exception exception) {
exception.printStackTrace();
}
return url;
}
public static String upload(MultipartFile file, String bizPath) throws IOException {
String fileName = file.getOriginalFilename();
String suffix = fileName.substring(fileName.lastIndexOf("."));
String key = bizPath + "/" + UUID.randomUUID().toString().replaceAll("-", "") + suffix;
ObsClient obsClient = new ObsClient(accessKey, secretKey, endpoint);
try {
obsClient.putObject(bucketName, key, file.getInputStream());
} finally {
obsClient.close();
}
return "https://" + bucketName + "." + endpoint + "/" + key;
}
```
5.调用upload方法上传图片到华为云OBS:
```java
MultipartFile file = ...; // 获取上传的文件
String bizPath = "images"; // 业务路径
String uploadType = CommonConstant.UPLOAD_TYPE_OBS; // 上传类型为OBS
String url = UploadUtil.upload(file, bizPath, uploadType); // 调用upload方法上传文件
System.out.println(url); // 输出上传后的文件URL
```
阅读全文
相关推荐
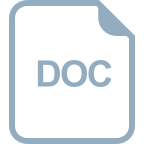
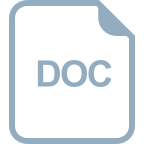
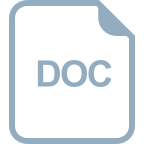

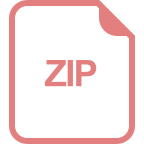
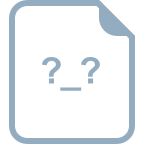
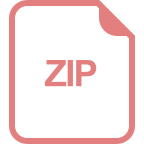





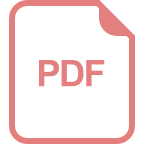
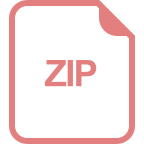
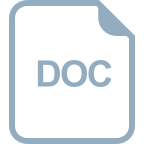
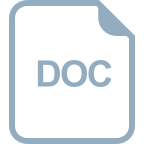
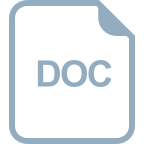