使用C编程从键盘输入一个小写英文字母,将其转换大写英文字母,将转换后的大写英文字母及其十进 制的ASCII码值显示到屏幕上
时间: 2024-09-30 14:15:16 浏览: 63
在C语言中,你可以使用标准库函数`toupper()`来进行字符大小写的转换,同时通过`printf()`函数将结果输出到屏幕。以下是一个简单的程序示例:
```c
#include <stdio.h>
#include <ctype.h> // 包含 toupper() 函数
int main() {
char ch; // 用于存储输入的小写字母
printf("请输入一个小写字母: ");
scanf("%c", &ch); // 从键盘读取输入
// 转换为大写
ch = toupper(ch);
// 输出大写字母和其ASCII码值
printf("转换后的字母是:%c\n", ch);
printf("ASCII码值是:%d\n", ch); // %d 是格式化字符串,用于打印整数
return 0;
}
```
在这个程序中,用户首先会被提示输入一个小写字母。然后,我们使用`toupper()`函数将输入的字符转换为大写,并利用`printf()`函数展示转换后的字符以及它的ASCII码。
相关问题
c语言编程从键盘输入一个小写英文字母,将其转换为大写英文字母,将转换后的大写英文字母及其十进制的ASCII码值显示到屏幕上。
在C语言中,可以使用ctype.h库中的`toupper()`函数将小写字母转换为大写字母,然后再使用`printf()`函数将结果以及对应的ASCII码值打印出来。下面是一个简单的示例:
```c
#include <stdio.h> // 包含标准输入输出库
#include <ctype.h> // 包含字符处理函数库
int main() {
char input_char, upper_case_char;
printf("请输入一个小写字母: ");
scanf("%c", &input_char); // 从键盘读取字符
// 转换为大写字母
upper_case_char = toupper(input_char);
// 打印转换后的字符和其ASCII码
printf("转换后的大写字母是: %c\n", upper_case_char);
printf("ASCII码值是: %d\n", (int)upper_case_char);
return 0;
}
```
当运行这个程序时,它会提示用户输入一个小写字母,然后将字母转换为大写,并同时显示出大写字母及对应的ASCII码。
c语言编程从键盘输入一个小写英文字母,将其转换为大写英文字母后,将转换后的大写英文字母及其十进制的ASCII码值显示到屏幕上
### 回答1:
```
#include <stdio.h>
int main() {
char lower;
printf("请输入一个小写字母:");
scanf("%c", &lower);
char upper = lower - 32; // 将小写字母转换为大写字母
printf("转换后的大写字母为:%c,ASCII码值为:%d\n", upper, upper);
return 0;
}
```
运行结果如下:
```
请输入一个小写字母:a
转换后的大写字母为:A,ASCII码值为:65
```
### 回答2:
C语言编程可以通过以下代码实现从键盘输入一个小写英文字母,将其转换为大写英文字母后,将转换后的大写英文字母及其十进制的ASCII码值显示到屏幕上:
```c
#include <stdio.h>
int main() {
char lowercase;
char uppercase;
printf("请输入一个小写英文字母:");
scanf("%c", &lowercase);
// 将小写字母转换为大写字母
uppercase = lowercase - 32;
printf("转换后的大写英文字母为:%c\n", uppercase);
printf("ASCII码值为:%d\n", uppercase);
return 0;
}
```
运行该程序后,会提示用户输入一个小写英文字母。用户输入后,将使用ASCII码表中大写字母与小写字母的差值(32)将其转换为大写字母,并分别将转换后的大写字母和对应的ASCII码值打印到屏幕上。
注意:该代码忽略了用户可能输入的非小写字母的情况,需添加相关的输入校验逻辑来确保输入合法。
### 回答3:
这是一个简单的C语言程序,用于输入一个小写英文字母,将其转换为大写英文字母,并显示转换后的大写英文字母及其十进制的ASCII码值。
```c
#include <stdio.h>
int main() {
char lowercase;
printf("请输入一个小写英文字母:");
scanf("%c", &lowercase);
// 将小写字母转换为大写字母
char uppercase = lowercase - 32;
printf("转换后的大写字母为:%c\n", uppercase);
printf("转换后的大写字母的ASCII编码为:%d\n", uppercase);
return 0;
}
```
程序先提示用户输入一个小写英文字母,然后通过`scanf`函数将输入的字符保存在`lowercase`变量中。接着,通过将`lowercase`变量减去32,将小写字母转换为大写字母,并保存在`uppercase`变量中。最后,通过`printf`函数分两次打印出转换后的大写字母及其十进制的ASCII码值。
注意,这个程序中没有对输入进行错误检查,所以如果用户输入了一个非小写英文字母,将导致程序输出的结果不准确。为了进一步完善程序,可以添加输入检查功能。
相关推荐
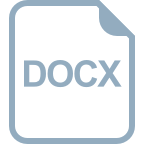
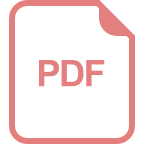
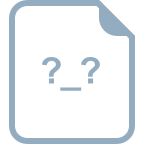













