XMLSlideShow转 MultipartFile
时间: 2023-12-21 10:32:04 浏览: 26
根据提供的引用内容,以下是将XMLSlideShow转换为MultipartFile的示例代码:
```java
import org.springframework.http.MediaType;
import org.springframework.http.codec.multipart.FilePart;
import org.springframework.http.codec.multipart.Part;
import org.springframework.http.codec.multipart.SynchronossPartHttpMessageReader;
import org.springframework.util.MultiValueMap;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestPart;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import reactor.core.publisher.Mono;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
@RestController
public class FileController {
@PostMapping("/api/convert")
public Mono<String> convertToMultipartFile(@RequestPart("file") FilePart filePart) throws IOException {
// 将XMLSlideShow转换为MultipartFile
MultipartFile multipartFile = convertXMLSlideShowToMultipartFile(filePart);
// 处理multipartFile文件逻辑
// ...
return Mono.just("success");
}
private MultipartFile convertXMLSlideShowToMultipartFile(FilePart filePart) throws IOException {
// 读取XMLSlideShow文件内容
Path tempFile = Files.createTempFile("temp", ".pptx");
filePart.transferTo(tempFile).block();
// 将文件内容转换为字节数组
byte[] fileBytes = Files.readAllBytes(tempFile);
// 创建MultipartFile对象
return new InMemoryMultipartFile(filePart.filename(), filePart.headers().contentType(), fileBytes);
}
private static class InMemoryMultipartFile implements MultipartFile {
private final String name;
private final MediaType contentType;
private final byte[] content;
public InMemoryMultipartFile(String name, MediaType contentType, byte[] content) {
this.name = name;
this.contentType = contentType;
this.content = content;
}
@Override
public String getName() {
return name;
}
@Override
public String getOriginalFilename() {
return name;
}
@Override
public String getContentType() {
return contentType.toString();
}
@Override
public boolean isEmpty() {
return content.length == 0;
}
@Override
public long getSize() {
return content.length;
}
@Override
public byte[] getBytes() throws IOException {
return content;
}
@Override
public InputStream getInputStream() throws IOException {
return new ByteArrayInputStream(content);
}
@Override
public void transferTo(Path dest) throws IOException, IllegalStateException {
Files.copy(getInputStream(), dest, StandardCopyOption.REPLACE_EXISTING);
}
}
}
```
请注意,上述代码是基于Spring Boot框架的示例,用于将XMLSlideShow转换为MultipartFile。在这个示例中,我们使用了Spring的`FilePart`类来处理文件上传,并使用自定义的`InMemoryMultipartFile`类来创建`MultipartFile`对象。
相关推荐
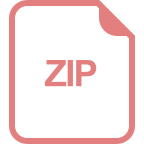














