Js interface
时间: 2024-08-27 14:01:00 浏览: 77
在JavaScript中,接口(Interface)是一种规范,它定义了一组方法和属性,但并不提供具体的实现。接口主要用于描述类的行为特征,而不是它的状态。通过接口,你可以确保某个类实现了你需要的功能,即使你不需要关心其实现细节。
创建一个接口使用`interface`关键字,比如:
```javascript
interface MyInterface {
sayHello(): string;
goodbye(): void;
}
```
在这个例子中,`MyInterface`定义了两个方法:`sayHello`返回字符串,和`goodbye`不返回值。任何想要被看作是这个接口的实现的类都需要实现这两个方法。例如:
```javascript
class MyClass implements MyInterface {
sayHello() {
return 'Hello!';
}
goodbye() {
console.log('Goodbye!');
}
}
let obj = new MyClass();
obj.sayHello(); // 输出 "Hello!"
obj.goodbye(); // 输出 "Goodbye!"
```
相关问题
javascript interface 调用
JavaScript Interface 是指 JavaScript 和 Native 代码之间的一个接口,通过这个接口可以实现 JavaScript 调用 Native 代码的功能。在 Android 平台上,我们可以通过在 Java 代码中定义一个带有 @JavascriptInterface 注解的方法,然后在 WebView 中使用 addJavascriptInterface 方法将这个 Java 对象注入到 JavaScript 环境中,从而实现 JavaScript 调用 Native 代码的功能。
下面是一个简单的示例代码:
在 Java 代码中定义一个带有 @JavascriptInterface 注解的方法:
```java
public class WebAppInterface {
Context mContext;
/** Instantiate the interface and set the context */
WebAppInterface(Context c) {
mContext = c;
}
/** Show a toast from the web page */
@JavascriptInterface
public void showToast(String toast) {
Toast.makeText(mContext, toast, Toast.LENGTH_SHORT).show();
}
}
```
在 WebView 中使用 addJavascriptInterface 方法将这个 Java 对象注入到 JavaScript 环境中:
```java
WebView webView = (WebView) findViewById(R.id.webview);
webView.addJavascriptInterface(new WebAppInterface(this), "Android");
```
在 JavaScript 中调用这个 Native 方法:
```javascript
<input type="button" value="Say hello" onClick="showAndroidToast('Hello Android!')" />
<script type="text/javascript">
function showAndroidToast(toast) {
Android.showToast(toast);
}
</script>
```
当用户点击按钮时,就会调用 showAndroidToast 方法,该方法调用 Android.showToast 方法,从而弹出一个 Toast。
interface js
As an AI language model, I do not have the capability to create interfaces in JavaScript. However, I can provide some information about interfaces in JavaScript.
In JavaScript, an interface is a description of the structure and behavior of an object. It specifies a set of properties and methods that an object must have in order to be considered to implement that interface. Interfaces are not enforced by the language itself, but they are useful for documenting and communicating the expected behavior of objects.
One way to define an interface in JavaScript is by using TypeScript, which is a superset of JavaScript that adds static typing and other features. TypeScript interfaces can be used to define the shape of objects and ensure that they have the required properties and methods.
Here is an example of a TypeScript interface that describes an object with a `name` property and a `greet` method:
```
interface Person {
name: string;
greet(): void;
}
```
To use this interface, you can define a class that implements it:
```
class Greeter implements Person {
name: string;
constructor(name: string) {
this.name = name;
}
greet() {
console.log(`Hello, ${this.name}!`);
}
}
const greeter = new Greeter('World');
greeter.greet(); // logs "Hello, World!"
```
This code defines a `Greeter` class that implements the `Person` interface. The class has a `name` property and a `greet` method that logs a greeting to the console. When you create a new instance of the `Greeter` class and call the `greet` method, it logs a message using the `name` property of the object.
While interfaces are not enforced by the language itself, they can be useful for ensuring that objects have the expected shape and behavior, and for communicating that behavior to other developers.
阅读全文
相关推荐
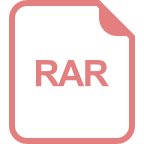
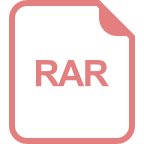
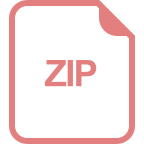
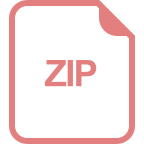
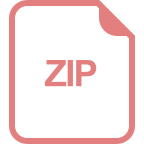
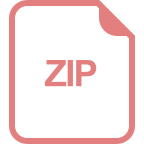
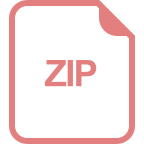
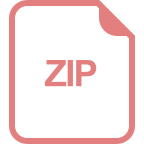
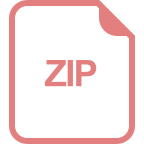
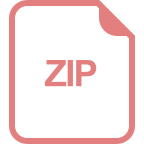
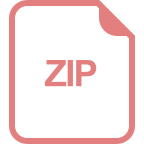




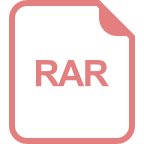