如何使用C#编程实现每月的最后一天自动执行某个任务?
时间: 2024-11-15 08:31:05 浏览: 16
在 C# 中,我们可以利用 `DateTime` 类的特性结合 `Task` 或 `Timer` 来编写一个函数,使其在每个月的最后一天自动执行某个任务。下面是一个简单的例子,使用了 `DateTime` 和 `Task.Delay`:
```csharp
using System;
using System.Threading.Tasks;
public class MonthlyExecution
{
private static Timer monthlyExecutionTimer;
public static void Start()
{
// 获取当前日期并初始化计时器
var currentDate = DateTime.Today;
monthlyExecutionTimer = new Timer(Task.Run, ScheduleExecution(currentDate), 0, MonthEndDelay(currentDate));
}
private static DateTime ScheduleExecution(DateTime currentDate)
{
// 计算下一个月的最后一天
return new DateTime(currentDate.Year, currentDate.Month, DateTime.DaysInMonth(currentDate.Year, currentDate.Month + 1), 23, 59, 59); // 设置为一天结束时间
}
private static int MonthEndDelay(DateTime currentDate)
{
// 返回本月剩余的时间到月末(小时*60+分钟)
return (int)(DateTime.Today.AddMonths(1) - currentDate).TotalHours * 60;
}
public static void ExecuteTask()
{
// 这里放置你要在每月最后一天执行的任务,比如打印一条消息
Console.WriteLine("Executing task on the last day of the month.");
}
public static void Cancel()
{
// 取消定时器,防止意外执行
monthlyExecutionTimer.Dispose();
}
public static void Main()
{
try
{
Start();
// 模拟运行几个月
for (int i = 0; i < 12; i++)
{
Thread.Sleep(1000); // 每秒检查一次是否到达每月最后一天
}
// 检查当前日期是否为最后一天
if (DateTime.Today.Day == DateTime.DaysInMonth(DateTime.Today.Year, DateTime.Today.Month))
{
ExecuteTask();
}
Cancel();
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
}
}
```
在这个示例中,`Start` 方法启动定时器,`ScheduleExecution` 函数计算下个月的最后一天,`MonthEndDelay` 计算本月剩余到月末的时间差。在 `Main` 中,我们模拟了一个运行过程,在实际应用中,你可能需要替换 `Thread.Sleep` 为更精确的检查机制。
阅读全文
相关推荐



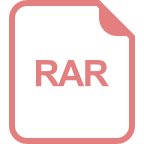

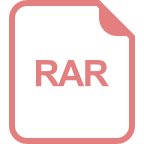



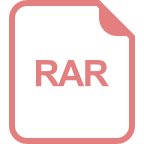
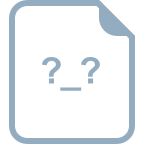

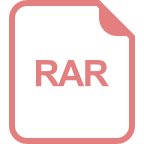





