用vue做一个记事本,记事本是一个数组对象,里面id和name两个,有初始数据,用vue实现添加和修改数据,并双向绑定
时间: 2024-10-17 14:09:34 浏览: 4
创建一个简单的Vue记事本应用,首先需要设置初始数据,然后实现添加、修改以及双向数据绑定。以下是步骤:
1. 创建一个新的Vue项目:
```bash
vue create simple-notepad-app
```
进入项目目录:
```bash
cd simple-notepad-app
```
2. 配置组件结构:
在`src/components`文件夹下创建`NoteList.vue` (用于展示列表) 和 `NoteInput.vue` (用于输入新内容)。
3. 添加数据和初始化:
在`NoteList.vue`中,设置初始数据并监听数组变化:
```html
<template>
<div>
<NoteInput v-on:add="addNote" />
<ul>
<li v-for="(note, index) in notes" :key="index">
<span>{{ note.id }}</span> - <span>{{ note.name }}</span>
<button @click="editNote(index)">编辑</button>
<button @click="deleteNote(index)">删除</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
notes: [
{ id: 1, name: '第一条记录' },
{ id: 2, name: '第二条记录' } // 初始数据
]
};
},
methods: {
addNote(note) {
this.notes.push(note);
},
editNote(index) {
const newNote = prompt("请输入新的内容");
if (newNote) {
this.notes[index].name = newNote;
}
},
deleteNote(index) {
this.notes.splice(index, 1);
}
}
};
</script>
```
4. 实现双向数据绑定:
在`NoteInput.vue`中,使用`v-model`绑定了输入框的值:
```html
<template>
<input v-model="inputText" placeholder="请输入新内容" @keyup.enter="submit" />
</template>
<script>
export default {
props: ['add'],
methods: {
submit() {
this.add && this.add({ id: Date.now(), name: this.inputText });
this.inputText = '';
}
}
};
</script>
```
5. 现在,当你在`NoteInput`中输入并按Enter键,或者在`NoteList`中点击“添加”按钮时,数据会在列表中增加,同时,列表和输入框都会实时更新。
阅读全文
相关推荐
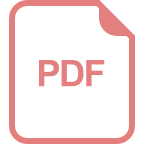
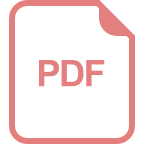
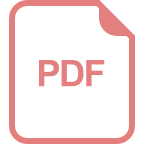















