使用【C语言】写【文本相似度分析】的代码将一段电文进行代替密码加密
时间: 2024-10-16 16:19:10 浏览: 35
在C语言中,文本相似度分析通常是用于比较两个字符串之间的相似度,而直接作为密码加密通常涉及更复杂的算法,如哈希函数、对称加密或公钥加密等。然而,如果你想要简单地替换电文中的字符(例如,替换每个字母),你可以使用凯撒密码(Caesar Cipher)的一个简单版本。下面是一个简单的凯撒密码替换示例:
```c
#include <stdio.h>
#include <string.h>
char shift(char c, int s) {
// ASCII表上A-Z和a-z的位置
const char offset = 'a';
if (c >= 'a' && c <= 'z') {
return ((c - offset + s) % 26) + offset;
} else if (c >= 'A' && c <= 'Z') {
return ((c - 'A' + s) % 26) + 'A';
}
// 如果不是字母,保持不变
return c;
}
void caesar_encrypt(const char* plaintext, char* ciphertext, int shift_amount) {
int len = strlen(plaintext);
for (int i = 0; i < len; ++i) {
ciphertext[i] = shift(plaintext[i], shift_amount);
}
ciphertext[len] = '\0'; // 添加终止符
}
int main() {
char plaintext[100];
printf("请输入明文:");
fgets(plaintext, sizeof(plaintext), stdin); // 要从用户输入读取
plaintext[strlen(plaintext) - 1] = '\0'; // 移除换行符
char ciphertext[100];
caesar_encrypt(plaintext, ciphertext, 3); // 示例:偏移量为3
printf("密文为:%s\n", ciphertext);
return 0;
}
```
这个程序使用了凯撒密码,其中`shift`函数负责字符替换,`caesar_encrypt`函数实现了整体加密过程。输入明文后,它会将每个字母向前移动指定的位数。
阅读全文
相关推荐
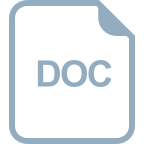
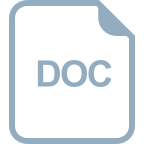
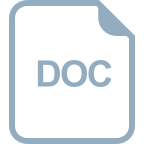
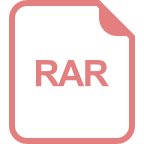
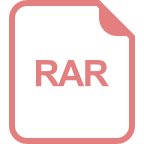
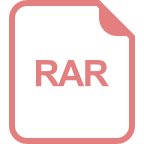
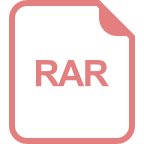
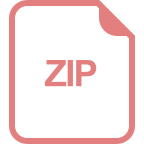
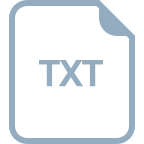
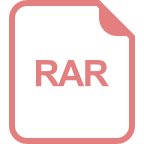
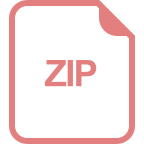
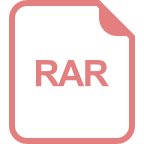