有没有具体的代码举例
时间: 2024-06-24 13:00:29 浏览: 93
当然可以,以下是一个简单的多态性示例:
```c++
#include<iostream>
using namespace std;
class Shape {
protected:
int width, height;
public:
Shape( int a = 0, int b = 0) {
width = a;
height = b;
}
virtual int area() {
cout << "Parent class area :" <<endl;
return 0;
}
};
class Rectangle: public Shape {
public:
Rectangle( int a = 0, int b = 0):Shape(a, b) { }
int area () {
cout << "Rectangle class area :" <<endl;
return (width * height);
}
};
class Triangle: public Shape{
public:
Triangle( int a = 0, int b = 0):Shape(a, b) { }
int area () {
cout << "Triangle class area :" <<endl;
return (width * height / 2);
}
};
int main() {
Shape *shape;
Rectangle rec(10,7);
Triangle tri(10,5);
// 存储矩形的地址
shape = &rec;
// 调用矩形的求面积函数 area
shape->area();
// 存储三角形的地址
shape = &tri;
// 调用三角形的求面积函数 area
shape->area();
return 0;
}
```
在这个例子中,我们定义了一个 `Shape` 基类,它有两个成员变量 `width` 和 `height`。然后我们又定义了两个子类 `Rectangle` 和 `Triangle`,分别继承了 `Shape`。这两个子类都重写了 `Shape` 的 `area()` 函数,因此它们的实现不同。在主函数中,我们首先定义了一个 `Shape` 类型的指针 `shape`,然后分别将 `Rectangle` 和 `Triangle` 对象的地址赋给它,最后调用 `area()` 函数。
这样做的好处是,在编译时无需知道具体对象类型,只需要知道对象的基类类型即可。这种方式可以让程序更加灵活、可扩展,并且代码更加简洁易懂。
阅读全文
相关推荐




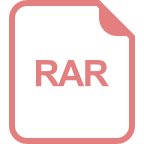

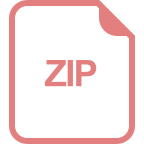
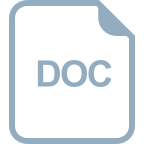







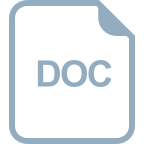