c语言读取csv文件的两列
时间: 2024-09-30 14:05:23 浏览: 36
在C语言中直接操作CSV文件通常是通过文件I/O函数完成的,由于C语言本身并不提供解析CSV的功能,我们通常会借助一些外部库,如`csv-parser`或者自己编写简单的解析算法。下面是一个基本的例子,展示了如何使用标准库读取CSV文件的两列数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体存储CSV行的数据
typedef struct {
char column1[50]; // 假设第一列为字符串类型
char column2[50]; // 假设第二列为字符串类型
} CsvRow;
// 解析一行CSV数据
CsvRow parse_csv_line(char* line) {
CsvRow row;
char* comma = strchr(line, ',');
if (comma) {
strncpy(row.column1, line, comma - line); // 获取第一列
*comma++ = '\0'; // 移除逗号
// 再次查找第二个逗号
comma = strchr(comma, ',');
if (comma) {
strncpy(row.column2, comma + 1, strlen(comma)); // 获取第二列并移除尾部换行符
*comma = '\0';
} else {
row.column2[0] = '\0'; // 如果只有一个字段,设置第二列为空
}
} else {
row.column1[0] = '\0'; // 如果只有一列
}
return row;
}
int main() {
FILE* file = fopen("example.csv", "r");
if (file == NULL) {
printf("Failed to open file\n");
return 1;
}
char buffer[1024];
while (fgets(buffer, sizeof(buffer), file)) {
CsvRow row = parse_csv_line(buffer);
printf("Column 1: %s, Column 2: %s\n", row.column1, row.column2);
}
fclose(file);
return 0;
}
阅读全文
相关推荐
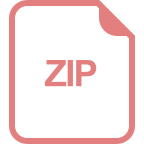
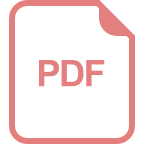

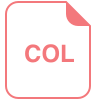


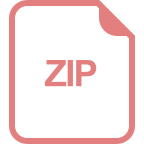
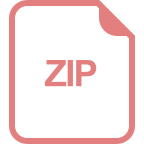
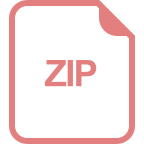
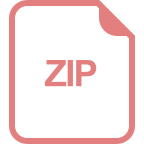
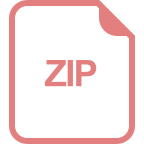
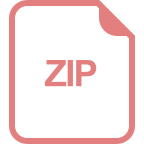
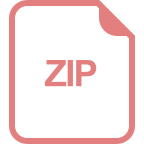
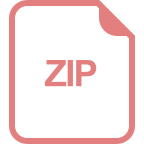
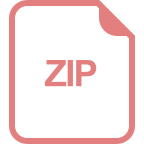
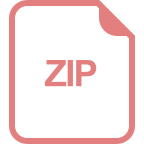
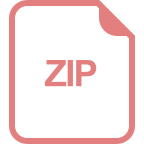