PhpSpreadsheet 把一个单元格拆分成两行两列并写入内容
时间: 2024-10-19 09:04:15 浏览: 22
PHP Spreadsheet是一个强大的库,用于读取、操作Excel文件,特别是在处理Microsoft Excel (.xls)和OpenDocument (.ods)格式上。如果你想要将一个单元格的内容拆分成两行两列并写入,你可以通过以下步骤来实现:
1. 首先,你需要加载已经存在的Excel工作簿或者创建一个新的工作簿。可以使用`PhpOffice\PhpSpreadsheet\IOFactory`来加载文件:
```php
use PhpOffice\PhpSpreadsheet\IOFactory;
$spreadsheet = IOFactory::load('your_file.xlsx');
```
2. 找到你想拆分的单元格。例如,假设你想要处理A1单元格,你可以获取它的`getActiveSheet()`并定位到这个位置:
```php
$cell = $spreadsheet->getActiveSheet()->getCell('A1');
```
3. 获取当前单元格的数据,通常用`getCellValue()`方法:
```php
$data = $cell->getValue();
```
4. 将数据分割成四部分,这里假设你是按照逗号分隔每一行和每一列:
```php
$rows = explode("\n", $data);
$columns = array_column(explode(',', current($rows)), 0); // 仅取第一列作为示例
```
5. 写入新的单元格。由于题目提到的是两行两列,我们可以用循环遍历数组并将内容分别写入对应的位置。例如,A2到B2和A3到B3:
```php
foreach ($columns as $index => $columnData) {
$rowIndex = 2 * ($index / 2) + 1; // 确定行号 (1-based)
$colIndex = floor($index % 2) + 2; // 确定列号 (从A开始)
$newCell = $spreadsheet->getActiveSheet()->getCellByColumnAndRow($colIndex, $rowIndex);
$newCell->setValue($columnData);
// 如果需要换行写入其他列,这里继续添加相应的行号
}
```
6. 最后,保存修改后的Excel文件:
```php
$writer = \PhpOffice\PhpSpreadsheet\Writer\Xlsx::create($spreadsheet);
$writer->save('output.xlsx');
```
阅读全文
相关推荐
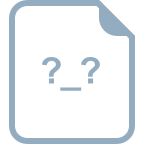
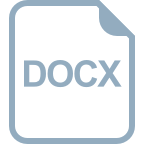

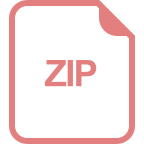
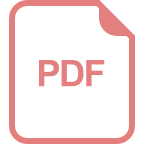
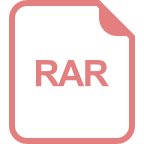
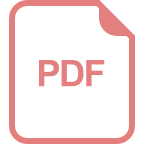
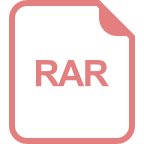
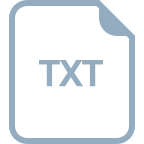
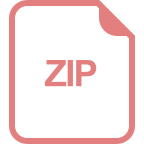
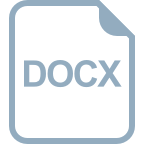
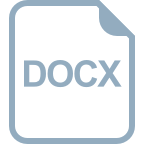
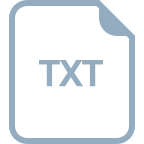
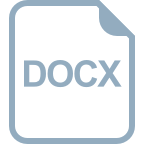
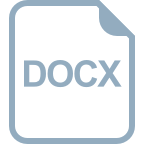
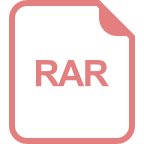