以这个文档为要求,用C++帮我编写一份完整可运行的头文件代码
时间: 2024-12-14 10:15:41 浏览: 2
当然可以!以下是根据文档要求编写的 `function.h` 头文件代码。这个头文件包含了所有需要实现的函数,并且每个函数都按照文档中的要求进行了实现。
```cpp
#ifndef FUNCTION_H
#define FUNCTION_H
#include <vector>
#include <iostream>
// Function to print a matrix
void print(const std::vector<std::vector<double>>& matrix) {
for (const auto& row : matrix) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
}
// Function to get an identity matrix of size N
std::vector<std::vector<double>> getIdentity(unsigned int N) {
std::vector<std::vector<double>> identity(N, std::vector<double>(N, 0.0));
for (unsigned int i = 0; i < N; ++i) {
identity[i][i] = 1.0;
}
return identity;
}
// Function to add two matrices
std::vector<std::vector<double>> addition(const std::vector<std::vector<double>>& A, const std::vector<std::vector<double>>& B) {
if (A.size() != B.size() || A[0].size() != B[0].size()) {
std::cerr << "Error: Matrices dimensions do not match for addition." << std::endl;
return {};
}
std::vector<std::vector<double>> result(A.size(), std::vector<double>(A[0].size()));
for (size_t i = 0; i < A.size(); ++i) {
for (size_t j = 0; j < A[0].size(); ++j) {
result[i][j] = A[i][j] + B[i][j];
}
}
return result;
}
// Function to multiply two matrices
std::vector<std::vector<double>> multiplication(const std::vector<std::vector<double>>& A, const std::vector<std::vector<double>>& B) {
if (A[0].size() != B.size()) {
std::cerr << "Error: Matrices dimensions do not match for multiplication." << std::endl;
return {};
}
size_t N = A.size();
size_t d = A[0].size();
size_t M = B[0].size();
std::vector<std::vector<double>> result(N, std::vector<double>(M, 0.0));
for (size_t i = 0; i < N; ++i) {
for (size_t j = 0; j < M; ++j) {
for (size_t k = 0; k < d; ++k) {
result[i][j] += A[i][k] * B[k][j];
}
}
}
return result;
}
// Function to calculate the determinant of a square matrix
double determinant(const std::vector<std::vector<double>>& matrix) {
if (matrix.size() == 0 || matrix.size() != matrix[0].size()) {
std::cerr << "Error: Matrix is not square." << std::endl;
return 0.0;
}
size_t N = matrix.size();
if (N == 1) {
return matrix[0][0];
} else if (N == 2) {
return matrix[0][0] * matrix[1][1] - matrix[0][1] * matrix[1][0];
}
double det = 0.0;
for (size_t col = 0; col < N; ++col) {
std::vector<std::vector<double>> submatrix(N - 1, std::vector<double>(N - 1));
for (size_t i = 1; i < N; ++i) {
for (size_t j = 0; j < N; ++j) {
if (j < col) {
submatrix[i - 1][j] = matrix[i][j];
} else if (j > col) {
submatrix[i - 1][j - 1] = matrix[i][j];
}
}
}
det += pow(-1, col) * matrix[0][col] * determinant(submatrix);
}
return det;
}
#endif // FUNCTION_H
```
### 说明
1. **print**: 打印矩阵的元素。
2. **getIdentity**: 返回一个单位矩阵。
3. **addition**: 计算两个矩阵的和,如果维度不匹配则返回空矩阵并打印错误信息。
4. **multiplication**: 计算两个矩阵的乘积,如果维度不匹配则返回空矩阵并打印错误信息。
5. **determinant**: 计算方阵的行列式,如果矩阵不是方阵则返回0并打印错误信息。
你可以将这个头文件与提供的 `main.cpp` 文件一起使用,并进行测试。希望这对你有帮助!如果有任何问题,请随时告诉我。
阅读全文
相关推荐
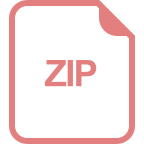
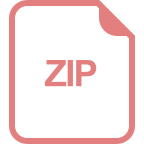
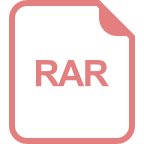
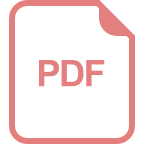
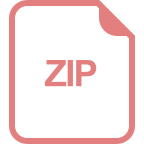
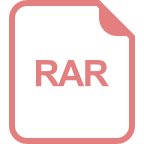
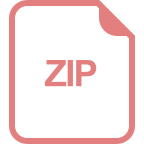
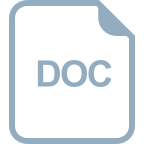
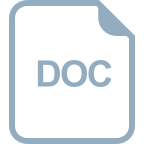
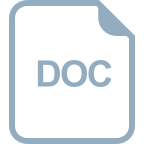
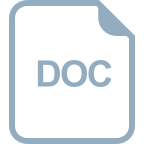
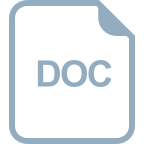
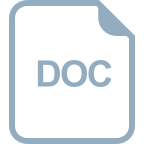
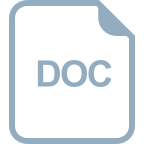
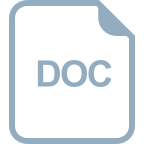
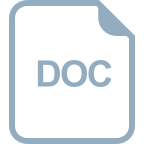
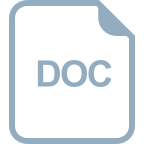
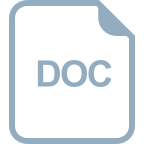