用C++编写一个程序,某学校的图书管理系统中,书本种类信息包括:书号、书名、价格三项属性,且书的种类数不超过20。要求使用双向循环链表表示。实现操作:初始化、插入、删除、从某一位置正序及倒叙输出所有书本信息(如4本书的情况下 按照2341、2143等顺序输出)。
时间: 2024-10-23 16:14:51 浏览: 79
在C++中,我们可以使用双向链表数据结构来实现这个图书管理系统的书籍信息存储。首先,我们需要定义一个Book节点类,包含书号、书名和价格属性,以及前驱和后继指针。然后,我们创建一个BookList类来管理整个链表。
```cpp
// 定义Book节点类
class Book {
public:
int bookID;
string title;
double price;
Book* prev; // 前驱指针
Book* next; // 后继指针
// 构造函数和析构函数省略
};
// 定义BookList类
class BookList {
private:
Book* head;
Book* tail;
public:
// 初始化链表
void init() {
head = new Book();
tail = head;
head->prev = nullptr;
tail->next = nullptr;
}
// 插入新书
void insert(Book* book) {
if (head == nullptr) {
head = tail = book;
book->prev = nullptr;
book->next = nullptr;
} else {
book->prev = tail;
book->next = nullptr;
tail->next = book;
tail = book;
}
if (book->bookID > 20) { // 确保种类数不超过20
cout << "Too many books, limit reached.\n";
return;
}
}
// 删除指定书本
void deleteBook(int bookID) {
Book* current = head;
while (current != nullptr) {
if (current->bookID == bookID) {
if (current == head) {
head = current->next;
if (head != nullptr)
head->prev = nullptr;
} else if (current == tail) {
tail = current->prev;
tail->next = nullptr;
} else {
current->prev->next = current->next;
current->next->prev = current->prev;
}
delete current;
break;
}
current = current->next;
}
}
// 正序输出所有书本信息
void printInOrder() {
Book* temp = head;
while (temp != nullptr) {
cout << temp->bookID << " - " << temp->title << " - $" << temp->price << endl;
temp = temp->next;
}
}
// 倒序输出所有书本信息
void printReversed() {
Book* temp = tail;
while (temp != nullptr) {
cout << temp->bookID << " - " << temp->title << " - $" << temp->price << endl;
temp = temp->prev;
}
}
};
int main() {
BookList system;
system.init();
// ... 进行书籍的插入、删除操作 ...
system.printInOrder(); // 正序输出
system.printReversed(); // 倒序输出
return 0;
}
```
阅读全文
相关推荐





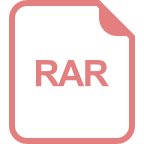
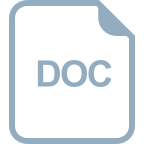
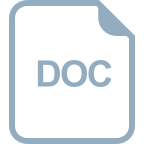

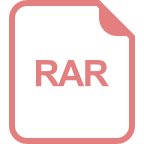
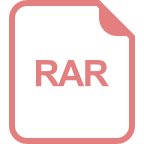
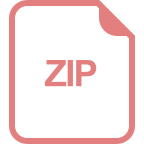
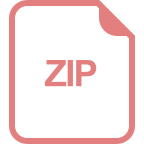