在C语言中,如何准确地实现一个函数来计算给定表达式 (sqrt(5) + sqrt(3)) / (-5 + sin(x)),并且特别地,当 x 被替换为 π/4 时应如何进行相应的数学运算?
时间: 2024-11-12 13:32:10 浏览: 14
在C语言中,实现这样一个函数需要引入一些库函数来处理数学运算,比如`<math.h>`库中的`sqrt()`和`sinf()`函数。首先,你需要包含这个头文件:
```c
#include <stdio.h>
#include <math.h>
```
接下来,你可以定义一个函数,例如`evaluate_expression(float x)`,来计算给定的表达式:
```c
double evaluate_expression(float x) {
double result = (sqrt(5.0) + sqrt(3.0)) / (-5.0 + sinf(x)); // 使用sinf()代替sin(x),因为它返回弧度值
return result;
}
```
当你想要计算 `x = π/4` 的时候,可以将这个值传递给这个函数:
```c
float pi_over_four = M_PI / 4; // 获取π/4的近似值
double expression_at_pi_quarter = evaluate_expression(pi_over_four);
printf("Expression at x = %f is: %.6f\n", pi_over_four, expression_at_pi_quarter);
```
相关问题
c语言计算 并输出表达式(b+ /√b²+2a)/(a-b )的值X。其中a和b的值从键盘输入代码
在C语言中,要计算并输出给定表达式的值,你需要使用数学库`math.h`来处理平方根运算,并使用`scanf`函数获取用户输入的a和b值。这里是一个简单的示例程序:
```c
#include <stdio.h>
#include <math.h>
int main() {
double a, b, result;
// 从键盘输入a和b的值
printf("请输入a的值: ");
scanf("%lf", &a);
printf("请输入b的值: ");
scanf("%lf", &b);
// 计算表达式 (b + √(b^2 + 2a)) / (a - b)
result = (b + sqrt(b * b + 2 * a)) / (a - b); // 注意使用sqrt()函数计算平方根
// 输出结果
if (a != b) { // 防止除数为零的情况
printf("表达式的结果是: %.2lf\n", result);
} else {
printf("警告:除数不能为0!\n");
}
return 0;
}
```
y=(根号x+2x)/x-5用c语言表达
在C语言中,表达式 `y = (sqrt(x) + 2*x) / x - 5` 可以通过函数 `sqrt()` 来计算平方根,并结合算术运算符 `/` 和 `-` 来实现。请注意,C语言标准库并没有内置的 `sqrt()` 函数,你需要包含 `<math.h>` 头文件并使用 `sqrt()` 函数从 `<math>` 库获取支持。以下是该表达式的C语言版代码:
```c
#include <stdio.h>
#include <math.h>
double calculate_y(double x) {
double root_x = sqrt(x);
double numerator = root_x + 2 * x;
double denominator = x;
double y = (numerator / denominator) - 5;
return y;
}
int main() {
double input_value;
printf("请输入一个数 x: ");
scanf("%lf", &input_value);
// 检查输入是否为正数,因为sqrt()函数只接受非负数
if (input_value >= 0) {
double result = calculate_y(input_value);
printf("y值为: %lf\n", result);
} else {
printf("输入错误,x必须是非负数。\n");
}
return 0;
}
```
在这个程序里,`calculate_y` 函数负责计算给定 `x` 的 `y` 值。
阅读全文
相关推荐
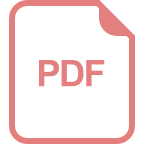
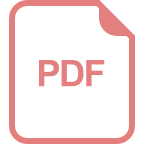

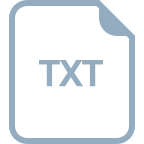
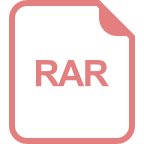
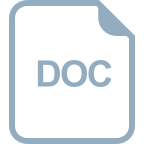
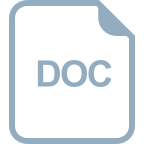
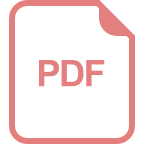
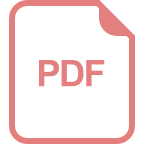
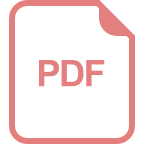
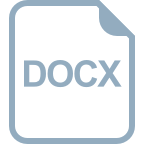
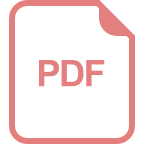
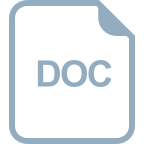
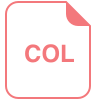


