react 函数组件刷新后清空localStorage
时间: 2024-10-20 11:16:33 浏览: 22
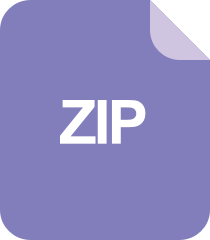
localstorage-in-react-hooks
React函数组件默认不会直接操作浏览器的localStorage。如果你想在刷新页面(即组件卸载后)清除localStorage的数据,你需要在组件的生命周期钩子中处理这个逻辑。这里可以使用`useEffect`钩子结合` localStorage.clear()`方法:
```jsx
import React, { useState, useEffect } from 'react';
function MyFunctionComponent() {
const [data, setData] = useState(() => window.localStorage.getItem('yourDataKey') || {}); // 获取或初始化localStorage数据
useEffect(() => {
// 当组件卸载(页面刷新)时清除localStorage
return () => {
localStorage.removeItem('yourDataKey'); // 清除指定键的数据
};
}, []);
// 其他组件逻辑...
return (
{/* 组件渲染内容 */}
);
}
export default MyFunctionComponent;
```
在这个例子中,我们首先使用`useState`获取或初始化存储的数据,然后在`useEffect`的返回函数里添加了一个清理逻辑。当组件卸载时,会自动触发这个清理步骤。
阅读全文
相关推荐
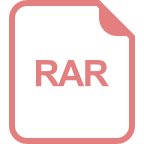
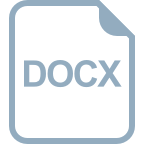
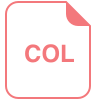


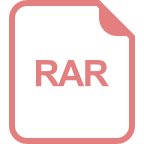
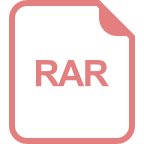
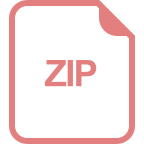
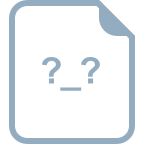
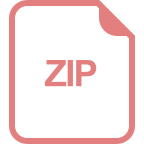
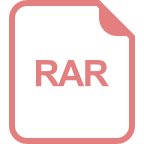
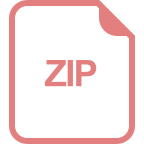
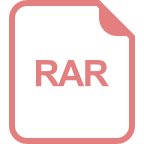
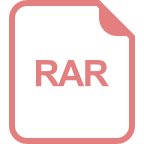