java中multipart/form-data设置参数
时间: 2023-10-13 19:04:14 浏览: 293
在Java中使用`multipart/form-data`格式发送请求并设置参数,可以使用Java的第三方库,例如Apache HttpClient或OkHttp。
下面是使用Apache HttpClient库的示例代码,演示如何设置`multipart/form-data`参数:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.entity.mime.content.StringBody;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import java.io.File;
import java.io.IOException;
public class MultipartFormDataExample {
public static void main(String[] args) {
String url = "http://example.com/upload";
File file = new File("file.txt");
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
HttpPost httpPost = new HttpPost(url);
// 创建多部分实体构造器
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
// 添加文件参数
FileBody fileBody = new FileBody(file, ContentType.DEFAULT_BINARY);
builder.addPart("file", fileBody);
// 添加其他参数
builder.addPart("param1", new StringBody("value1", ContentType.TEXT_PLAIN));
builder.addPart("param2", new StringBody("value2", ContentType.TEXT_PLAIN));
// 构建多部分实体
HttpEntity multipartEntity = builder.build();
// 设置请求实体
httpPost.setEntity(multipartEntity);
// 发送请求并获取响应
HttpResponse response = httpClient.execute(httpPost);
// 处理响应
System.out.println(response.getStatusLine());
// 其他处理逻辑...
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
上述示例中,我们使用`MultipartEntityBuilder`构建`multipart/form-data`请求体,添加文件参数和其他参数。最后,将构建的请求实体设置到`HttpPost`对象中,并发送请求。
请注意,示例中使用的是Apache HttpClient 4.x版本。如果你使用的是不同版本的HttpClient库,请根据库的文档进行相应调整。
希望对你有帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
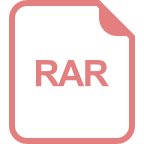
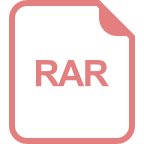







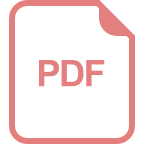
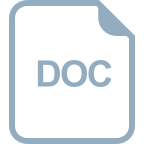






