用c++将结构体类型的数据存入一个二进制文件
时间: 2024-10-28 15:08:44 浏览: 26
在C++中,你可以使用`std::ofstream`和成员访问运算符`.`来将结构体类型的数据存入二进制文件。这里是一个简单的步骤:
首先,定义一个结构体(struct):
```cpp
#include <fstream>
// 定义一个结构体
struct Person {
std::string name;
int age;
double salary;
};
```
然后,创建一个函数来写入数据到二进制文件:
```cpp
void writeBinaryFile(const Person& person, const char* filename) {
std::ofstream outfile(filename, std::ios::binary);
if (outfile.is_open()) {
// 使用sizeof获取每个成员的字节数,然后写入
outfile.write(reinterpret_cast<const char*>(&person.name), sizeof(person.name));
outfile.write(reinterpret_cast<const char*>(&person.age), sizeof(person.age));
outfile.write(reinterpret_cast<const char*>(&person.salary), sizeof(person.salary));
outfile.close();
std::cout << "Data written to file successfully.\n";
} else {
std::cerr << "Failed to open the file for writing.\n";
}
}
```
最后,你可以通过创建Person对象并调用这个函数来保存它:
```cpp
int main() {
Person p {"Alice", 30, 50000.0};
writeBinaryFile(p, "data.bin");
return 0;
}
```
阅读全文
相关推荐
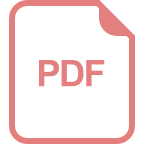
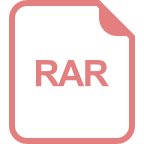
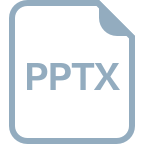


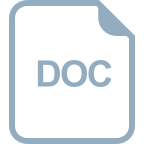
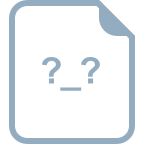
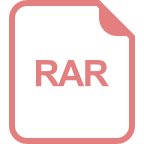
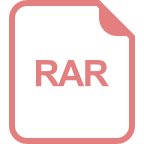
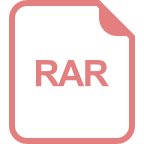
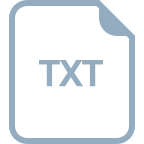
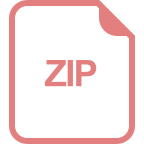
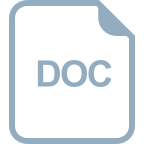
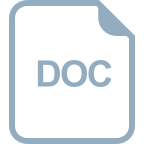
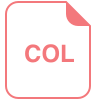
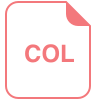
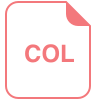
