Element wise multiplication
时间: 2024-08-15 17:05:17 浏览: 34
Element-wise multiplication, often referred to as the Hadamard product, is a binary operation that takes two equal-sized vectors or matrices and produces another vector or matrix of the same size. For each corresponding element in the input arrays, it multiplies them together to produce an element in the output array.
### Basic Concept
In simpler terms, if you have two arrays (or matrices) `A` and `B`, their element-wise multiplication results in a new array `C` where each element `c[i][j]` is calculated as follows:
```
c[i][j] = a[i][j] * b[i][j]
```
where `a[i][j]` and `b[i][j]` are elements at position `[i][j]` in `A` and `B` respectively.
### Example with Vectors
For instance, consider two simple vectors `A = [4, 5, 6]` and `B = [2, 3, 4]`. Their element-wise multiplication would yield:
```
[4*2, 5*3, 6*4] = [8, 15, 24]
```
### Usage in Programming
Element-wise multiplication is commonly used in various fields such as mathematics, statistics, computer science, particularly in machine learning for operations on tensors in frameworks like TensorFlow, PyTorch, NumPy, etc. It's useful for performing calculations on data points across multiple dimensions simultaneously.
### Benefits
1. **Efficiency**: For large datasets, element-wise operations can be significantly faster than equivalent looping constructs because they take advantage of optimized numerical libraries.
2. **Conciseness**: They allow complex mathematical operations to be written in fewer lines of code.
3. **Parallel Processing**: They are easily parallelizable, making them suitable for high-performance computing scenarios.
### Implementation in Python with NumPy
Here’s how you might perform an element-wise multiplication using Python and the NumPy library:
```python
import numpy as np
# Define your arrays
array_A = np.array([4, 5, 6])
array_B = np.array([2, 3, 4])
# Perform element-wise multiplication
result = np.multiply(array_A, array_B)
print(result)
```
### Related Questions
1. **What are some applications of element-wise multiplication?**
- How does it simplify complex algorithms?
- Can you provide examples beyond basic math?
2. **How does element-wise multiplication differ from matrix multiplication?**
- What are the implications for computational efficiency?
3. **Can element-wise operations be applied to non-numerical data types?**
- If so, under what conditions do these operations make sense?
相关推荐
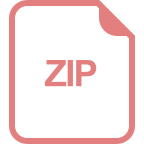
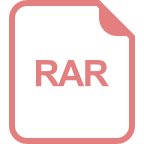
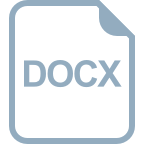














