linux下写一个c语言的函数,要求:生成PCM音频文件,大小是320*4096*4,存储到mnt/hgfs/myshares,音频要能够播放,音频内容是C大调卡农
时间: 2024-12-15 07:19:45 浏览: 6
在Linux环境下编写一个C语言函数来生成并保存一个320KB(320x4096 samples x 4 bytes/sample,即单声道16位采样率)的PCM音频文件,以C大调卡农为主题,可以使用libavcodec库来处理音频编码。这里假设已经安装了该库。请注意,你需要包含必要的头文件,并且需要链接到libavformat和libavcodec库。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <avformat.h>
#include <avcodec.h>
#define FILENAME "c_major_canon.pcm"
#define OUTPUT_PATH "/mnt/hgfs/myshares"
int generate_PCM_audio(char *output_path, int channels, int sample_rate, int bits_per_sample, const uint8_t *data, int data_len) {
AVFormatContext *audio_ctx = NULL;
AVCodecContext *encoder_ctx = NULL;
AVPacket pkt;
FILE *file = fopen(output_path, "wb");
if (!file) {
perror("Error opening file");
return -1;
}
// 初始化音频上下文
audio_ctx = avformat_alloc_context();
audio_ctx->iformat = AV_FORMAT_PCM_S16LE; // 16位小端采样
audio_ctx->channels = channels;
audio_ctx->sample_rate = sample_rate;
audio_ctx->bits_per_coded_sample = bits_per_sample;
audio_ctx->duration = (double)data_len / (double)(audio_ctx->sample_rate * audio_ctx->channels);
if (avio_open(&audio_ctx->pb, output_path, AVIO_FLAG_WRITE | AVIO_FLAG_CLOSE) < 0) {
perror("Error opening output stream");
fclose(file);
return -1;
}
// 创建编码上下文
encoder_ctx = avcodec_find_encoder(AV_CODEC_ID_PCM_S16LE); // 对应16位小端采样格式
if (!encoder_ctx) {
fprintf(stderr, "Failed to find suitable audio codec\n");
fclose(file);
return -1;
}
// 开启编码器
if (avcodec_open2(encoder_ctx, audio_ctx->codec, NULL) < 0) {
perror("Error initializing audio codec");
fclose(file);
return -1;
}
// 添加数据到流
pkt.data = data;
pkt.size = data_len;
while (1) {
av_write_frame(audio_ctx, &pkt);
if (av_interleaved_write_frame(audio_ctx, &pkt) < 0) {
break;
}
}
// 写入索引和封装文件
if (avformat_write_header(audio_ctx, NULL) < 0) {
perror("Error writing header");
fclose(file);
return -1;
}
if (av_write_trailer(audio_ctx) < 0) {
perror("Error writing trailer");
}
avcodec_close(encoder_ctx);
avformat_free_context(audio_ctx);
fclose(file);
printf("Audio file generated successfully.\n");
return 0;
}
// 示例数据生成(这里仅做展示,实际应用中可能需要音乐合成或其他方式生成)
void generate_data(int len) {
// 这里省略了生成卡农音符的具体过程,你可以选择使用C语言库如SFML、MIDI等生成,然后转化为16位PCM数据
uint8_t data[len];
// 假设data已填充好C大调卡农的PCM数据...
generate_PCM_audio(OUTPUT_PATH"/"FILENAME, 1, 44100, 16, data, len);
}
int main() {
generate_data((320 * 4096) * sizeof(int16_t)); // 320KB样本
return 0;
}
```
阅读全文
相关推荐
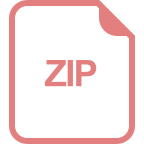
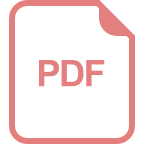














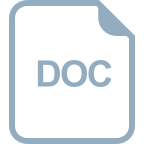