c++中如何重载赋值运算符
时间: 2024-06-17 14:07:33 浏览: 25
在C++中,重载赋值运算符是一种特殊的函数,它用于将一个对象的值赋给另一个对象。它的语法形式如下:
```cpp
class MyClass {
public:
// 重载赋值运算符
MyClass& operator=(const MyClass& other) {
// 进行赋值操作
return *this;
}
};
```
其中,`MyClass` 是一个类名,`operator=` 是关键字,表示重载赋值运算符,`other` 是要赋值的对象。
在重载赋值运算符时,需要注意以下几点:
1. 重载赋值运算符的返回类型应该是该类的引用,即 `MyClass&`。
2. 重载赋值运算符的参数应该是一个常量引用,即 `const MyClass&`。
3. 在函数体内,需要将传入的对象的值赋给当前对象,并返回当前对象。
下面是一个示例:
```cpp
#include <iostream>
class MyClass {
public:
MyClass(int value = 0) : m_value(value) {}
// 重载赋值运算符
MyClass& operator=(const MyClass& other) {
if (this != &other) { // 防止自我赋值
m_value = other.m_value;
}
return *this;
}
int getValue() const { return m_value; }
private:
int m_value;
};
int main() {
MyClass obj1(100);
MyClass obj2(200);
obj1 = obj2; // 调用重载的赋值运算符
std::cout << obj1.getValue() << std::endl; // 输出 200
return 0;
}
```
相关推荐
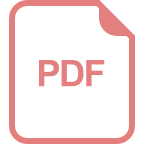
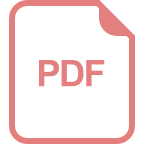














