vue 拖拽排序组件
时间: 2023-08-25 15:07:45 浏览: 53
你可以使用vue-draggable组件来实现拖拽排序的功能。这是一个基于Vue.js的拖拽排序组件,可以帮助你方便地实现列表的拖拽排序。
首先,你需要安装vue-draggable组件。在命令行中运行以下命令来安装:
```
npm install vuedraggable
```
安装完成后,在你的Vue组件中引入该组件:
```javascript
import draggable from 'vuedraggable'
```
接下来,你可以在你的模板中使用`draggable`组件来实现拖拽排序:
```html
<draggable v-model="list">
<div v-for="item in list" :key="item.id">{{ item.name }}</div>
</draggable>
```
在上面的例子中,`list`是一个包含了要排序的数据对象的数组。通过`v-model`指令将`list`与`draggable`组件进行绑定,使其能够响应拖拽并更新数据。
你可以根据自己的需求进行样式和功能的定制,比如添加拖拽时的占位符、限制拖拽的方向等。更多详细的用法和配置选项可以参考vue-draggable的官方文档。
希望以上信息对你有帮助!如果还有其他问题,请随时提问。
相关问题
vue3实现组件拖拽排序
Vue3 的组件拖拽排序可以通过使用 `Sortable.js` 库来实现。以下是一个简单的示例:
1. 安装 `Sortable.js` 库:
```
npm install sortablejs --save
```
2. 在需要排序的组件中导入 `Sortable.js`:
```javascript
import Sortable from 'sortablejs';
```
3. 在组件的 `mounted` 钩子中初始化排序:
```javascript
mounted() {
const el = this.$refs.sortable;
this.sortable = Sortable.create(el, {
onEnd: this.onSortEnd
});
},
```
4. 创建 `onSortEnd` 方法来处理排序结束后的逻辑:
```javascript
methods: {
onSortEnd(event) {
const itemEl = event.item;
const newIndex = event.newIndex;
// 处理排序结束后的逻辑
}
}
```
5. 在组件中添加拖拽排序的 HTML:
```html
<template>
<div ref="sortable">
<div v-for="(item, index) in items" :key="item.id">
{{ item.text }}
</div>
</div>
</template>
```
在以上代码中,`items` 是一个数组,包含需要排序的项目。将 `items` 数组绑定到组件的属性中即可。
完整的代码示例:
```javascript
<template>
<div ref="sortable">
<div v-for="(item, index) in items" :key="item.id">
{{ item.text }}
</div>
</div>
</template>
<script>
import Sortable from 'sortablejs';
export default {
data() {
return {
items: [
{ id: 1, text: 'Item 1' },
{ id: 2, text: 'Item 2' },
{ id: 3, text: 'Item 3' },
{ id: 4, text: 'Item 4' }
],
sortable: null
};
},
mounted() {
const el = this.$refs.sortable;
this.sortable = Sortable.create(el, {
onEnd: this.onSortEnd
});
},
methods: {
onSortEnd(event) {
const itemEl = event.item;
const newIndex = event.newIndex;
// 处理排序结束后的逻辑
}
}
};
</script>
```
写一个vue拖动组件排序
好的,这里是一个简单的 Vue 拖拽排序组件的示例代码。这个组件可以让用户通过拖拽列表项来重新排序它们。
```vue
<template>
<div>
<ul>
<li v-for="(item, index) in list" :key="item.id" :draggable="true"
@dragstart="dragStart(index)" @dragover="dragOver(index)"
@dragend="dragEnd">
{{ item.text }}
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
list: [
{ id: 1, text: "Item 1" },
{ id: 2, text: "Item 2" },
{ id: 3, text: "Item 3" },
{ id: 4, text: "Item 4" },
{ id: 5, text: "Item 5" }
],
draggingItem: null
};
},
methods: {
dragStart(index) {
this.draggingItem = this.list[index];
},
dragOver(index) {
if (this.draggingItem === null) {
return;
}
const draggingIndex = this.list.indexOf(this.draggingItem);
if (index !== draggingIndex) {
this.list.splice(draggingIndex, 1);
this.list.splice(index, 0, this.draggingItem);
}
},
dragEnd() {
this.draggingItem = null;
}
}
};
</script>
```
这个组件的实现基于 HTML5 的拖放 API。在模板中,我们使用 `v-for` 指令来渲染列表项,并为每个列表项绑定拖放事件。在 `dragStart` 方法中,我们记录正在拖拽的列表项。在 `dragOver` 方法中,我们根据拖拽位置重新排序列表项。最后,在 `dragEnd` 方法中,我们清空正在拖拽的列表项。
相关推荐
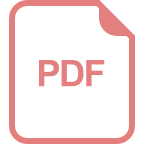
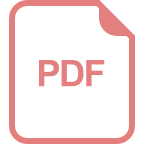
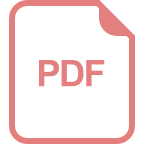












